Restarting (Recycling) an Application Pool
Solution 1
If you're on IIS7 then this will do it if it is stopped. I assume you can adjust for restarting without having to be shown.
// Gets the application pool collection from the server.
[ModuleServiceMethod(PassThrough = true)]
public ArrayList GetApplicationPoolCollection()
{
// Use an ArrayList to transfer objects to the client.
ArrayList arrayOfApplicationBags = new ArrayList();
ServerManager serverManager = new ServerManager();
ApplicationPoolCollection applicationPoolCollection = serverManager.ApplicationPools;
foreach (ApplicationPool applicationPool in applicationPoolCollection)
{
PropertyBag applicationPoolBag = new PropertyBag();
applicationPoolBag[ServerManagerDemoGlobals.ApplicationPoolArray] = applicationPool;
arrayOfApplicationBags.Add(applicationPoolBag);
// If the applicationPool is stopped, restart it.
if (applicationPool.State == ObjectState.Stopped)
{
applicationPool.Start();
}
}
// CommitChanges to persist the changes to the ApplicationHost.config.
serverManager.CommitChanges();
return arrayOfApplicationBags;
}
If you're on IIS6 I'm not so sure, but you could try getting the web.config and editing the modified date or something. Once an edit is made to the web.config then the application will restart.
Solution 2
Here we go:
HttpRuntime.UnloadAppDomain();
Solution 3
Maybe this articles will help:
- Recycle current Application Pool programmatically (for IIS 6+)
- Recycling Application Pools using WMI in IIS 6.0
- Recycling IIS 6.0 application pools programmatically
- Programatically recycle an IIS application pool
Solution 4
The code below works on IIS6. Not tested in IIS7.
using System.DirectoryServices;
...
void Recycle(string appPool)
{
string appPoolPath = "IIS://localhost/W3SVC/AppPools/" + appPool;
using (DirectoryEntry appPoolEntry = new DirectoryEntry(appPoolPath))
{
appPoolEntry.Invoke("Recycle", null);
appPoolEntry.Close();
}
}
You can change "Recycle" for "Start" or "Stop" also.
Solution 5
I went a slightly different route with my code to recycle the application pool. A few things to note that are different than what others have provided:
1) I used a using statement to ensure proper disposal of the ServerManager object.
2) I am waiting for the application pool to finish starting before stopping it, so that we don't run into any issues with trying to stop the application. Similarly, I am waiting for the app pool to finish stopping before trying to start it.
3) I am forcing the method to accept an actual server name instead of falling back to the local server, because I figured you should probably know what server you are running this against.
4) I decided to start/stop the application as opposed to recycling it, so that I could make sure that we didn't accidentally start an application pool that was stopped for another reason, and to avoid issues with trying to recycle an already stopped application pool.
public static void RecycleApplicationPool(string serverName, string appPoolName)
{
if (!string.IsNullOrEmpty(serverName) && !string.IsNullOrEmpty(appPoolName))
{
try
{
using (ServerManager manager = ServerManager.OpenRemote(serverName))
{
ApplicationPool appPool = manager.ApplicationPools.FirstOrDefault(ap => ap.Name == appPoolName);
//Don't bother trying to recycle if we don't have an app pool
if (appPool != null)
{
//Get the current state of the app pool
bool appPoolRunning = appPool.State == ObjectState.Started || appPool.State == ObjectState.Starting;
bool appPoolStopped = appPool.State == ObjectState.Stopped || appPool.State == ObjectState.Stopping;
//The app pool is running, so stop it first.
if (appPoolRunning)
{
//Wait for the app to finish before trying to stop
while (appPool.State == ObjectState.Starting) { System.Threading.Thread.Sleep(1000); }
//Stop the app if it isn't already stopped
if (appPool.State != ObjectState.Stopped)
{
appPool.Stop();
}
appPoolStopped = true;
}
//Only try restart the app pool if it was running in the first place, because there may be a reason it was not started.
if (appPoolStopped && appPoolRunning)
{
//Wait for the app to finish before trying to start
while (appPool.State == ObjectState.Stopping) { System.Threading.Thread.Sleep(1000); }
//Start the app
appPool.Start();
}
}
else
{
throw new Exception(string.Format("An Application Pool does not exist with the name {0}.{1}", serverName, appPoolName));
}
}
}
catch (Exception ex)
{
throw new Exception(string.Format("Unable to restart the application pools for {0}.{1}", serverName, appPoolName), ex.InnerException);
}
}
}
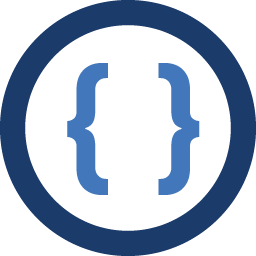
Admin
Updated on July 17, 2022Comments
-
Admin almost 2 years
How can I restart(recycle) IIS Application Pool from C# (.net 2)?
Appreciate if you post sample code?
-
DOK over 15 yearsOh, go ahead and show him how to adjust for restarting. Do you know how to do it?
-
Marc Gravell almost 15 yearsThat recycles the application, but I'm not sure it recycles the entire app-pool (which can host multiple applications at once).
-
Nathan Ridley about 13 years@Marc - very valid, though sometimes you only care about the current application context. The conditions indicating the need to reload may assert themselves independently in each instance.
-
ThisGuyKnowsCode almost 13 yearsVery helpful, I've been needing this! (I only need it for the current context)
-
ashes999 over 12 years+1 you are the man. After no less than 10 solutions (including touching web.config), this was the winnar.
-
Gabriel about 12 yearsNote that you need to have "IIS 6 WMI Compatibility" enabled on IIS7
-
Jon almost 12 yearsHi, this is a very old post but I am struggling to figure one part out. Where does "ServerManagerDemoGlobals.ApplicationPoolArray" come from? i.e. what should I reference to access it? I have added references to Microsoft.Web.Management.dll and Microsoft.Web.Administration.dll Thanks
-
Holger almost 12 years@Jon I'm also having that problem.
-
Holger almost 12 years@Jon The code seem to originate from here: msdn.microsoft.com/en-us/library/…
-
Simply G. over 10 yearsThis especially if you have Unknown Error 0x80005000 from trying one of the other solutions. That are all good in their own way.
-
Simply G. over 10 yearsI recommend this on how to make your own 'ExecuteDosCommand' method. codeproject.com/Articles/25983/How-to-Execute-a-Command-in-C
-
Baahubali about 10 yearsHow would you run the above command if you are running it on a remote server?
-
Brad Irby over 9 yearsI was able to satisfy most references by adding the NuGet package Microsoft.Web.Administration. I still cannot find the PropertyBag class. MSDN says it's in Microsoft.Web.Management.Server but I can't find that dll anywhere. Anybody know where it is?
-
Brad Irby over 9 yearsI added a reference to this and it satisfied the attribute and propertyBag c:\windows\SysWOW64\inetsrv\Microsoft.Web.Management.dll (also found in c:\windows\system32\inetsrv\Microsoft.Web.Management.dll)
-
Royi Namir over 9 years+1 Im just curious , why would anyone want to recycle his App ? I mean what are the scenarious ( im an asp.net ) developer.
-
Mox Shah over 9 yearsI'm getting an error at line
ApplicationPoolCollection applicationPoolCollection = serverManager.ApplicationPools;
Filename: redirection.config Error: Cannot read configuration file due to insufficient permissions -
sirdank over 9 years@Jon Unless you need to use the ArrayList to pass on your app pool object, just delete the code and don't sweat it. It's only needed because of the example it appears in on MSDN.
-
Aaron Hudon almost 9 yearsVery useful if you need to remotely recycle your web app (e.g. long-lived / singleton objects are misbehaving)
-
peter about 8 yearswhere the hell is ServerManagerDemoGlobals.ApplicationPoolArray??
-
BornToCode almost 8 years@DOK - To adjust that code for restarting (recycle) the application pool, change:
applicationPool.Start();
ToapplicationPool.Recycle();
and remove theif (applicationPool.State == ObjectState.Stopped)
condition. -
sairfan almost 7 yearsworks good on my iis8, error free just need to add reference Microsoft.Web.Administration as mentioned.
-
Viacheslav Shchupak about 6 yearsThis one worked the best for me. Except that I made 2 calls: stop and start instead on recycle
-
Jimbo almost 5 years@RoyiNamir - a bit of a necro-post but, if one wanted to clear all session data (for all users) this appears to be the only way to do that.