Retrieving column names with column values in sqlite using cursor
12,277
Solution 1
In this case you should use hashmap
for (int i =0 ; i< count; i++)
{
String data = cursor.getString(i);
String column_name = cursor.getColumnName(i);
HashMap<String,String> map = new HashMap<String,String>();
map.put("column_value",data);
map.put("column_name",column_name);
details.add(map); //change the type of details from ArrayList<String> to arrayList<HashMap<String,String>>
}
Solution 2
Use getColumnName(int columnIndex)
to get the name of the row for a given index.
Solution 3
Use this.
for (int i =0 ; i< count; i++)
{
String data = cursor.getString(i);
String column_name = cursor.getColumName(i);
details.add(data);
}
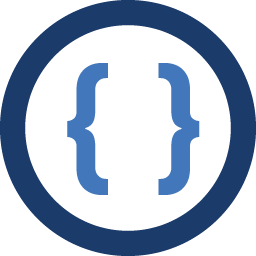
Author by
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I want to display column names with column values in the Listview using cursor. My present code shows only the column values.
public void openAndQueryDatabase() { db = openOrCreateDatabase( "mydatabase.db", SQLiteDatabase.CREATE_IF_NECESSARY , null ); Cursor cursor = db.rawQuery("select * from "+ table + " where name='"+ name + "'", null); int count = cursor.getColumnCount(); if (cursor!=null ) { if (cursor.moveToFirst()) { do { for (int i =0 ; i< count; i++) { String data = cursor.getString(i); details.add(data); } } while (cursor.moveToNext()); } } }