Return value using button
Solution 1
Try taking a look at this link https://stackoverflow.com/a/11907627/3110529 as it addresses the issues you're having.
The main concern is that callbacks are designed to just be a behaviour that happens in response to an event and therefore it doesn't entirely make sense for them to return a value. In your program, when you say
if bla == 1:
print('1')
you're asking if the function pointer (or reference?) is equal to 1
which it obviously will never be.
You might be tempted to use global variables to cover this (i.e. store a 'blah' variable, then have the call back set its value) but this is generally considered bad practice. Instead, as in the link, try converting it to a class which will allow you to use member variables to store the results and responses of callbacks in a more organised manner.
Solution 2
Your problem is that you arn't saving the return value of bla()
.
You can do this using a global variable:
from Tkinter import *
master = Tk()
master.geometry("200x100")
gloBla = 0
def bla():
global gloBla
gloBla = 1
Button(master, command = bla, text = 'OK').pack()
mainloop()
if gloBla == 1:
print('1')
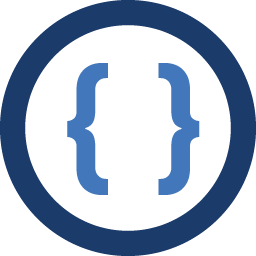
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I'm trying to return a value using a button, but i just can't get it to work. What i want is the button to return a value which later on can be used to check is the function was used or not. The code that I'm using is the following:
from Tkinter import * master = Tk() master.geometry("200x100") def bla(): return 1 Button(master, command = bla, text = 'OK').pack() if bla == 1: print('1') mainloop()
I've also tried to do this using lambda but i could not figure that one out too.
-
Readme almost 8 yearsThis is actually better than the answer i wrote. Here, have my upvote.