Returning integer array from function with no arguments
Solution 1
Pretend you don't know what C-arrays are and join the world of modern C++:
#include <array>
std::array<int, 8> getNums()
{
std::array<int, 8> ret = {{ 1, 2, 3, 4, 5, 6, 7, 8 }};
return ret;
}
If your compiler is too old to provide a std::
or std::tr1::
implementation of array<>
, consider using boost::array<>
instead. Or, consider using std::vector<>
either way.
Solution 2
I am led understand that when the function ends the pointer is lost, but will the array still be sent?
The behavior is undefined.
what is a good way to return this integer array with no arguments in the function call?
int nums[8];
num
is local variable which resides on stack. You cannot return the reference of a local variable. Instead alloc nums
with operator new
and remember to delete[]
it.
int* getNums()
{
int *nums = new int[8] ;
// .....
return nums ;
}
// You should deallocate the resources nums acquired through delete[] later,
// else memory leak prevails.
Solution 3
Whenever a function exits all the local variables created within that function get trashed.
You are creating an array local to the function and then returning a pointer to the array. The returned pointer will point to an memory location which is already reclaimed by the OS. So it wont work for you.
Instead of Arrays, You should use vectors, since it is C++
Solution 4
You can't return a simple array in C++. Try
int *getNums()
{
int *nums = new int[8];
...
return nums;
}
Now nums
is a pointer to a heap array which will live on after getNums
returns.
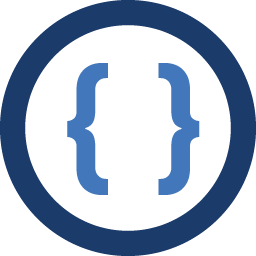
Admin
Updated on June 12, 2022Comments
-
Admin almost 2 years
Possible Duplicate:
Returning local data from functions in C and C++ via pointerI need to create a function with no arguments that returns an array
I get the error: "warning: function returns address of local variable"
my code has been simplified for ease of reading
int * getNums() { int nums[8]; nums = {1,2,3,4,5,6,7,8}; return nums; }
I am led understand that when the function ends the pointer is lost, but will the array still be sent? If not, what is a good way to return this integer array with no arguments in the function call?
Appreciate the help in advance!
Cheers
-
sbi almost 13 years
-1
from me for storing anew
'd object in a naked pointer. -
sbi almost 13 yearsStoring a
new
'd object in a naked pointer is a very bad idea, and your warning is not enough to make up for that. -
Mahesh almost 13 years@sbi - Could you please elaborate your comment so that I can correct.
-
zoul almost 13 yearsSorry, I started with a C solution, than noticed the C++ tag and ventured into an unknown territory. I’ll return back to the plain C example I am comfortable with :) Thank you for the correction, what exactly can go wrong in that case?
-
Kiran Kumar almost 13 years@Mahesh: Instead of doing manual memory management you can use smart pointers such as
boost::shared_array
in these cases. -
Mahesh almost 13 years@Naveen - I was just hinting the OP as how to return an array. I am not well acquainted with boost library and any how thanks for your comment.
-
Matthieu M. almost 13 years@Mahesh: the issue is one of resources (memory here) management. Using a naked pointer you put the onus on the caller wrt correctly returning the memory once she's done with it. It is mandatory to use a proper resources manager (usually a smart pointer), otherwise you get brittle code (and probably leaky one). In C++03, you'd use a
std::vector<int>
. -
Mahesh almost 13 years@Matthieu M. - At least I should have hinted OP to use
std::vector
in my answer. I was just thinking in terms of his question and answered. In general, is it advisable for beginners to usestd::vector
at all ? I believe, one should get strong hold as how to manage memory themselves and then start the usage of containers. -
Mike Seymour almost 13 yearsIf
std/tr1/boost::array
isn't available, then you could return a struct containing the array; that may be more efficient thanstd::vector
, particularly for small arrays. -
Matthieu M. almost 13 years@Mahesh: Since naked pointers are not to be used in C++, I would say it's rather available to teach idiomatic C++ from the start.
-
sbi almost 13 yearsIf you get a naked pointer from a function, you don't know whether you need to deallocate whatever it points to, and if you know, you don't know how. (Is it an object or an array? And was it
new
d ormalloc
'd?) And whenever you juggle with a dumb pointer to a resource you need to cleanup you're not exception-safe. For that you should use smart pointers, preferably off-the-shelf ones likestd::vector
orstd::shared_array
.