Reverse-Mapping for String Enums
Solution 1
We can make the Mode
to be a type and a value at the same type.
type Mode = string;
let Mode = {
Silent: "Silent",
Normal: "Normal",
Deleted: "Deleted"
}
let modeStr: string = "Silent";
let mode: Mode;
mode = Mode[modeStr]; // Silent
mode = Mode.Normal; // Normal
mode = "Deleted"; // Deleted
mode = Mode["unknown"]; // undefined
mode = "invalid"; // "invalid"
A more strict version:
type Mode = "Silent" | "Normal" | "Deleted";
const Mode = {
get Silent(): Mode { return "Silent"; },
get Normal(): Mode { return "Normal"; },
get Deleted(): Mode { return "Deleted"; }
}
let modeStr: string = "Silent";
let mode: Mode;
mode = Mode[modeStr]; // Silent
mode = Mode.Normal; // Normal
mode = "Deleted"; // Deleted
mode = Mode["unknown"]; // undefined
//mode = "invalid"; // Error
String Enum as this answer:
enum Mode {
Silent = <any>"Silent",
Normal = <any>"Normal",
Deleted = <any>"Deleted"
}
let modeStr: string = "Silent";
let mode: Mode;
mode = Mode[modeStr]; // Silent
mode = Mode.Normal; // Normal
//mode = "Deleted"; // Error
mode = Mode["unknown"]; // undefined
Solution 2
The cleanest way I found so far is to make a secondary map:
let reverseMode = new Map<string, Mode>();
Object.keys(Mode).forEach((mode: Mode) => {
const modeValue: string = Mode[<any>mode];
reverseMode.set(modeValue, mode);
});
Thus you can do let mode: Mode = reverseMode.get('Silent');
Advantages: no need to repeat the values, provides a way to enumerate the enum, keeps TSLint happy...
Edit: I originally write Mode[mode]
but then TS might throw the error TS7015 on this line, so I added the cast.
Solution 3
If you're ok with using Proxies, I made a more generic & compact version:
stringEnum.ts
export type StringEnum<T extends string> = {[K in T]: K}
const proxy = new Proxy({}, {
get(target, property) {
return property;
}
})
export default function stringEnum<T extends string>(): StringEnum<T> {
return proxy as StringEnum<T>;
}
Usage:
import stringEnum from './stringEnum';
type Mode = "Silent" | "Normal" | "Deleted";
const Mode = stringEnum<Mode>();
Solution 4
This answer is from @PhiLho,
I dont have enough rep to comment on his message.
let reverseMode = new Map<string, Mode>();
Object.keys(Mode).forEach((mode: Mode) => {
const modeValue: string = Mode[<any>mode];
reverseMode.set(modeValue, mode);
});
However, the first argument for .set
should be the key, and the second should be the value.
reverseMode.set(modeValue, mode);
should be...
reverseMode.set(mode, modeValue);
Resulting in this...
let reverseMode = new Map<string, ErrorMessage>();
Object.keys(ErrorMessage).forEach((mode: ErrorMessage) => {
const modeValue: string = ErrorMessage[<any>mode];
reverseMode.set(mode, modeValue);
});
The reason why @PhiLho might have missed this is because the keys and values for the original object provided by OP are the same.
Solution 5
The standard types achieves this function - just check for undefined and handle however.
type Mode = "Silent" | "Normal" | "Deleted";
const a = Mode["something else"];
//a will be undefined
Also, you can add on a record type to the above to extend the "enum", say to translate a short enum key into more verbose output (https://www.typescriptlang.org/docs/handbook/utility-types.html#recordkeys-type):
const VerboseMode: Record<Mode, string> = {
Silent: "Silent mode",
Normal: "Normal mode",
Deleted: "This thing has been deleted"
}
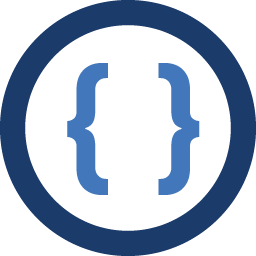
Admin
Updated on January 24, 2022Comments
-
Admin over 2 years
I wanted to use string enums in typescript but I can't see a support for reversed mapping in it. I have an enum like this:
enum Mode { Silent = "Silent", Normal = "Normal", Deleted = "Deleted" }
and I need to use it like this:
let modeStr: string; let mode: Mode = Mode[modeStr];
and yes I don't know what is it there in
modeStr
string and I need it parsed to the enum or a fail at parsing in runtime if the string is not presented in the enum definition. How can I do that as neat as it can be? thanks in advance -
Admin almost 7 yearsThanks...It could've been implemented in the typescript though.
-
omni over 6 yearstslint will throw an error on that Enum example: Element implicitly has an 'any' type because index expression is not of type 'number'. I guess the issue is that in TS string Enums can not be reverse-mapped, see the comment in the String-Enum example at typescriptlang.org/docs/handbook/release-notes/… - This seems to be true for TS 2.4 where String-Enum was introduced but I also get the error in TS 2.6.2
-
Rodris over 6 years@masi Since 2.4, TS has support for string enums, so you don't need to add the
<any>
cast. -
omni over 6 years@RodrigoPedrosa I'm aware of that. The error occues with and without the <any> cast. There's a GIT issue open related to that problem (github.com/Microsoft/TypeScript/issues/9234) but it was closed as "working as intended". Seems like TS devs never used any other lang that supports String-Evals otherwise they wouldn't claim it works as intended. At best it works "as good as possible" for a lang that tries to bring types to an other lang (I have not checked the tech. reasons so far thought).
-
Rodris over 6 years@masi I am not sure about what you are saying. The reverse mapping works in the playground. Is your problem specifically with the tslint?
-
omni over 6 years@RodrigoPedrosa Yes, it's a tslint issue. I'm not sure if the resulting code is working in all cases (typesafe). Example:
enum Mode {Silent = "Silent"}; let mode: Mode = Mode["Silent"];
will produce the error I posted in tslint. -
ofavre almost 4 yearsWe loose the compiler check in a switch when we cover all the cases though, and we must add a default case that throws (for instance when the compiler checks that all paths return a value), which in turn does not help by showing unhandled paths when we add new values. It's sad that TypeScript enums are so weird.