Riverpod Flutter: Looking for best way to combine FutureProvider with StateNotifierProvider
- You can fetch your ticketlist in your
TicketListNotifier
and set its state with your ticket list.
class TicketListNotifier extends RP.StateNotifier<List<Ticket>> {
TicketListNotifier() : super([]);
Future<void> fetchTicketList() async {
FirestoreService.getTicketList().when(
//success
// state = fetched_data_from_firestore
// error
// error handle
)
}
}
final ticketsController =
RP.StateNotifierProvider<TicketListNotifier, List<Ticket>>(
(ref) => TicketListNotifier(),
);
- Call this method where you want to fetch it /*maybe in your widget's initState method
ref.read(ticketsController.notifier).fetchTicketList();
Now ref.read(ticketsController);
will return your ticket list
- Since you have the ticket list in your TicketListNotifier's state you can use your add/remove method like this:
ref.read(ticketsController.notifier).addTicket(someTicket);
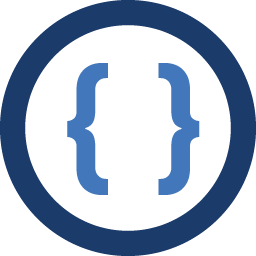
Admin
Updated on January 04, 2023Comments
-
Admin over 1 year
I am working on a basic Support Ticket System. I get the Tickets from Firebase (Either as a Stream or Future). I want to allow some Filtering Options (e.g. sort by Status and Category). For this, I thought about using A Future Provider to get the List and a StateNotiferProvider to update the List depending on which filter is being used. This is the code I have so far:
final ticketListStreamProvider = RP.FutureProvider((_) => FirestoreService.getTicketList()); class TicketListNotifier extends RP.StateNotifier<List<Ticket>> { TicketListNotifier() : super([]); void addTicket(Ticket ticket) { state = List.from(state)..add(ticket); } void removeTicket(Ticket ticket) { state = List.from(state)..remove(ticket); } } final ticketsController = RP.StateNotifierProvider<TicketListNotifier, List<Ticket>>( (ref) => TicketListNotifier(), );
There are multiple issues I have with that. Firstly it doesn't work. The StateNotifier accepts a List and not a Future<List>. I need to convert it somehow or rewrite the StateNotifier to accept the Future. I was trying to stay close to one of the official examples. (https://github.com/rrousselGit/riverpod/tree/master/examples/todos) Unfortunately, they don't use data from an outside source like firebase to do it.
What's the best approach to get resolve this issue with which combination of providers?
Thanks
-
Admin about 2 yearsThank you very much!
-
Admin about 2 yearsFollow-up question: While filtering I remove Tickets from the original list. When the user deselects a filter I need to add the filtered Tickets back in. Should I use another Provider to just always give me the Original list or is there a neater way to accomplish it?
-
Minjin Gelegdorj about 2 yearsYou can use both provider and setState() method. For example: ` List<Ticket> ticketList = ref.read(ticketsController); ...onClick(ticket) { setState()=> ticketList.remove(ticket); } `