Robot Framework using Python, key press without selecting any button or element in the page
Solution 1
If you want to send a key press event to the page, but not at a specific element - target the <body>
element in the page, using the Press Key
keyword:
Press Key xpath=//body \ue00f # this is the Page Down key
Press Key css=body \ue00e # and this - Page Up
An up-to-date list of key codes can be found in selenium's source, the webdriver.common.Keys module holds their definition.
IMHO libraries that execute keystrokes in the OS (like AutoIt, or Java's robot) should be avoided if possible - they make your automation scripts dependent on the execution machine (and operating system), and local only - you can't run browsers tests against Selenium Hub (Sauce labs, Browsertstack, similar).
Solution 2
Linux Alternative can be PyAutoGUI
Although it works for Windows and MacOS(cross-platform) also, as described in the docs
PyAutoGUI works on Python 2 & 3. Install from PyPI with
pip install pyautogui
Examples:
import pyautogui
def send_keys():
"""
Works on Windows/Mac/Linux
"""
pyautogui.press('enter') #Presses enter
pyautogui.hotkey('ctrl', 'shift', 'esc') #Performs ctrl+shift+esc
pyautogui.typewrite('Hello world!\n', interval=secs_between_keys) #Useful for entering text, newline is Enter
pyautogui.typewrite(['a', 'b', 'c', 'left', 'backspace', 'enter', 'f1'], interval=.4) #A list of key names can be passed too
Solution 3
Robot Framework Selenium library can only send keypresses to an element. If you want to send actual keypresses, you need to write your own library that does it. In Windows this can be done using SendKeys module.
Here is a library that defines "Send Enter Key" keyword for Robot Framework. I tested it quickly on Chrome, it might have problems with PhantomJS.
import SendKeys
def send_enter_key():
"""
Sends ENTER key to application
Works only in Windows
"""
SendKeys.SendKeys("{ENTER}")
Solution 4
Try it with two slashes, not three:
Press Key text_field q
Press Key login_button \\13 # ASCII code for the Enter key
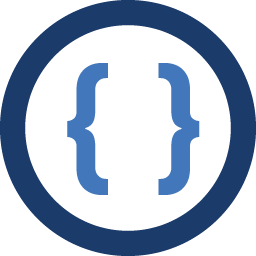
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I am automating one application using Robot Framework using Python. In a certain situation I need to press Enter without selecting any button or element of the page once the page is loaded.
I have tried with the below example (using
Press Key
), but it didn't work as I don't want to select any specific button or element of the page before press Enter on the page.Examples:
Press Key text_field q Press Key login_button \\\13 # ASCII code for the Enter key
The below keyword is not recognized by the IDE, most probably because of version:
Press Key Native
Is there a solution to get rid of this problem?