rotate a UIView around its center but several times
Solution 1
You can use the following animation on your UIView's layer property. I've tested it.
Objective-C
UIView *viewToSpin = ...;
CABasicAnimation* spinAnimation = [CABasicAnimation
animationWithKeyPath:@"transform.rotation"];
spinAnimation.toValue = [NSNumber numberWithFloat:5*2*M_PI];
[viewToSpin.layer addAnimation:spinAnimation forKey:@"spinAnimation"];
Swift 5.0
let viewToSpin = UIView() // However you have initialized your view
let spinAnimation = CABasicAnimation.init(keyPath: "transform.rotation")
spinAnimation.toValue = NSNumber(value: 5.0 * 2.0 * Float.pi)
viewToSpin.layer.add(spinAnimation, forKey: "spinAnimation")
Solution 2
As Brad Larson indicated, you can do this with a CAKeyframeAnimation
. For instance,
CAKeyframeAnimation *rotationAnimation;
rotationAnimation =
[CAKeyframeAnimation animationWithKeyPath:@"transform.rotation.z"];
rotationAnimation.values = [NSArray arrayWithObjects:
[NSNumber numberWithFloat:0.0 * M_PI],
[NSNumber numberWithFloat:0.75 * M_PI],
[NSNumber numberWithFloat:1.5 * M_PI],
[NSNumber numberWithFloat:2.0 * M_PI], nil];
rotationAnimation.calculationMode = kCAAnimationPaced;
rotationAnimation.removedOnCompletion = NO;
rotationAnimation.fillMode = kCAFillModeForwards;
rotationAnimation.timingFunction =
[CAMediaTimingFunction functionWithName:kCAMediaTimingFunctionEaseInEaseOut];
rotationAnimation.duration = 10.0;
CALayer *layer = [viewToSpin layer];
[layer addAnimation:rotationAnimation forKey:@"rotationAnimation"];
You can control the duration of the total animation with the rotationAnimation.duration
property, and the acceleration and deceleration (and calculation of steps in between) with the rotationAnimation.timingFunction
property.
Solution 3
Getting a continuous spinning effect is a little tricky, but I describe a means to do it here. Yes, Core Animation seems to optimize transforms to the closest ending position within the unit circle. The method I describe there chains a few half-rotation animations together to make full rotations, although you do notice a slight stutter in the handoff from one animation to the next.
Perhaps a CAKeyframeAnimation constructed with these half-rotation values would be the right way to go. Then you could also control acceleration and deceleration.
Solution 4
CABasicAnimation* animation = [CABasicAnimation animationWithKeyPath:@"transform.rotation.z"];
animation.fromValue = [NSNumber numberWithFloat:0.0f];
animation.toValue = [NSNumber numberWithFloat: 2*M_PI];
animation.duration = 8.0f;
animation.repeatCount = INFINITY;
[self.myView.layer addAnimation:animation forKey:@"SpinAnimation"];
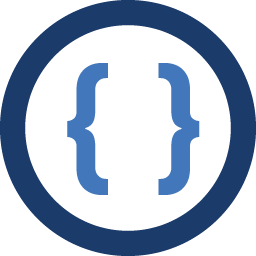
Admin
Updated on August 27, 2020Comments
-
Admin over 3 years
I'm trying to rotate some
UIView
around its center, so the simple code goes something like (in pseudocode):[UIView beginAnimations:@"crazyRotate" context:nil]; [UIView setAnimationDuration:1.0]; someview.transform = CGAffineTransformMakeRotation(angle); [UIView commitAnimations]
now if I set angle to say M_PI/2 the thing rotates nicely. if I set it to 2*M_PI, well it does "nothing". I can understand that the matrix translates to something that does nothing (rotating 360 means "stay" in a sense), yet, I want to rotate it 5 times (think of a newspaper rotate scale coming at you effect -- I'm not great at describing, hope someone understands). So, I tried adding setting angle to 180 deg (M_PI) and add a nested
animatationBlock
. but I guess that since I'm setting the same property (someview.transition
) again it ignores it somehow). I tried setting repeat count of the animation to 2 with angle M_PI but it seems to simply rotate 180, going back to straight position and then initiating the rotate again.So, I'm a little out of ideas, any help appreciated! --t
-
mahboudz almost 15 yearsHow can I slow down this animation?
-
Jason Medeiros over 14 yearsFor animation timing considerations, please see the reference material for the CAMediaTiming Protocol, which CABasicAnimation implements. Particularly, you'd probably want to set the 'duration' property. The code above is using the default duration of 0.25 seconds.