Round an answer to 2 decimal places in Python
Solution 1
You should use a format specifier:
print("6 Month Cost: %.2fUSD" % (cost * .6))
Even better, you shouldn't rely on floating point numbers at all and use the decimal
module instead, which gives you arbitrary precision and much more control over the rounding method:
from decimal import Decimal, ROUND_HALF_UP
def round_decimal(x):
return x.quantize(Decimal(".01"), rounding=ROUND_HALF_UP)
cost = Decimal(input("Enter 12 month cost: "))
print("6 Month Cost: ", round_decimal(cost * Decimal(".6")))
Solution 2
If you just want to have it as a string, format can help:
format(cost, '.2f')
This function returns a string that is formated as defined in the second parameter. So if cost contains 3.1418 the code above returns the string '3.14'.
Solution 3
A classic way is to multiply by 100, add 0.5 (this is to round) and int() the result. Now you have the number of rounded cents, divide by 100 again to get back the rounded float.
cost = 5.5566
cost *= 100 # cost = 555.66
cost += 0.5 # cost = 556.16
cost = int(cost) # cost = 556
cost /= float(100) # cost = 5.56
cost = 5.4444
cost = int(( cost * 100 ) + 0.5) / float(100) # cost = 5.44
Solution 4
If you just want it to print, string formatting will work:
print("\n12 Month Cost:%.2f USD"%(cost*1))
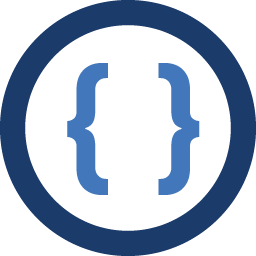
Admin
Updated on June 09, 2022Comments
-
Admin almost 2 years
The issue i am having is my rounding my results to 2 decimal places. My app gets the right results, however, i am having difficulty making the app round to the nearest decimal as you would with currency
cost = input("\nEnter the 12 month cost of the Order: ") cost = float(cost) print("\n12 Month Cost:", cost * 1,"USD") print("6 Month Cost:", cost * 0.60,"USD") print("3 Month Cost:", cost * 0.36,"USD")
so for example if the 12 month price is $23, the 6 month price is 13.799999999999999 but i want it to show 13.80
I've looked around google and how to round a number but couldn't find much help on rounding a result.
-
jvriesem over 9 yearsTaking this beyond currency formats, wouldn't it generally have better performance if we used a format specifier on regular Python decimal numbers than to use the
decimal
module?