Rounded corners cardView don't work in RecyclerView - Android?
Solution 1
It turns out that calling View.setBackgroundColor(int)
on a CardView
removes the rounded corners.
To change the background colour of a card and retain the corners, one needs to call CardView.setCardBackgroundColor(int)
instead.
That may be the case for certain visitors to this post.
When subclassing CardView
, I suggest adding the following method to protect your corners from accidental removal:
/**
* Override prevents {@link View#setBackgroundColor(int)} being called,
* which removes the rounded corners.
*/
@Override
public void setBackgroundColor(@ColorInt int backgroundColor) {
setCardBackgroundColor(backgroundColor);
}
In particular, I was working on a custom view implementation for React Native, and React was automatically applying a background colour to the view. This override solved that problem; it means other developers do not need to know the details of the underlying view.
Solution 2
use this in topmost layout declaration in xml layout file:
xmlns:card_view="http://schemas.android.com/apk/res-auto"
This solved the problem for me.
Solution 3
Try adding this two attributes in your card view
card_view:cardPreventCornerOverlap="false"
card_view:cardUseCompatPadding="true"
Here is the documentation for the first attribute (which contains reference to the second) https://developer.android.com/reference/android/support/v7/widget/CardView.html#setPreventCornerOverlap(boolean)
This should do the trick
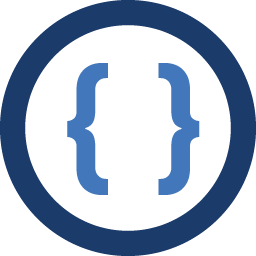
Admin
Updated on June 26, 2022Comments
-
Admin about 2 years
My android device is
4.3
and don't work round corner ofcardView
:<android.support.v7.widget.CardView xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/tools" xmlns:card_view="http://schemas.android.com/apk/res-auto" android:id="@+id/CardStart" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="5dp" android:layout_marginLeft="20dp" android:layout_marginRight="20dp" android:scaleType="centerCrop" app:cardUseCompatPadding="true" card_view:cardBackgroundColor="@color/BlackTrans" card_view:cardCornerRadius="5dp" card_view:cardElevation="0dp"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical"> <TextView android:id="@+id/txtTitle" android:layout_width="match_parent" android:layout_height="wrap_content" /> <TextView android:id="@+id/txtDescription" android:layout_width="match_parent" android:layout_height="wrap_content" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical"> <ImageButton android:id="@+id/imgbIcon" android:layout_width="wrap_content" android:layout_height="match_parent" android:src="@drawable/ic_serch" /> </LinearLayout> </LinearLayout> </android.support.v7.widget.CardView>
And I write bellow code in my class but don't work yet :
if (android.os.Build.VERSION.SDK_INT < Build.VERSION_CODES.LOLLIPOP) { holder.CardStart.setCardElevation(0); holder.CardStart.setBackgroundColor(ContextCompat.getColor(context,R.color.BlackTrans)); holder.CardStart.setRadius(5); holder.CardStart.setUseCompatPadding(true); }
-
kinsley kajiva almost 5 yearsthis works, i applied the method to my code and worked as expected.
-
Kedar Paranjape almost 5 yearsVery easy to make this mistake!
-
X09 over 3 yearsThanks, man! Found this after pulling my hair for almost 30 mins.