RSA encryption/decryption compatible with Javascript and PHP
Solution 1
Try the following simple example.
It is using a open source javascript library https://github.com/ziyan/javascript-rsa
HTML/JAVASCRIPT:
<script language="JavaScript" type="text/javascript" src="jsbn.js"></script>
<script language="JavaScript" type="text/javascript" src="rsa.js"></script>
<script language="JavaScript">
function encryptData(){
//Don't forget to escape the lines:
var pem="-----BEGIN PUBLIC KEY-----\
MIGfMA0GCSqGSIb3DQEBAQUAA4GNADCBiQKBgQDfmlc2EgrdhvakQApmLCDOgP0n\
NERInBheMh7J/r5aU8PUAIpGXET/8+kOGI1dSYjoux80AuHvkWp1EeHfMwC/SZ9t\
6rF4sYqV5Lj9t32ELbh2VNbE/7QEVZnXRi5GdhozBZtS1gJHM2/Q+iToyh5dfTaA\
U8bTnLEPMNC1h3qcUQIDAQAB\
-----END PUBLIC KEY-----";
var key = RSA.getPublicKey(pem);
element=document.getElementById('password');
element.value=RSA.encrypt(element.value, key);
}
</script>
<form method='POST' id='txtAuth' onsubmit='encryptData()'>
<input type='text' name='username'/>
<input type='password' name='password' id='password' placeholder="password"/>
<input name='submit' type='submit' value='Submit'>
</form>
PHP:
<?php
if (isset($_POST['password'])) {
//Load private key:
$private = "-----BEGIN RSA PRIVATE KEY-----
MIICXAIBAAKBgQDfmlc2EgrdhvakQApmLCDOgP0nNERInBheMh7J/r5aU8PUAIpG
XET/8+kOGI1dSYjoux80AuHvkWp1EeHfMwC/SZ9t6rF4sYqV5Lj9t32ELbh2VNbE
/7QEVZnXRi5GdhozBZtS1gJHM2/Q+iToyh5dfTaAU8bTnLEPMNC1h3qcUQIDAQAB
AoGAcbh6UFqewgnpGKIlZ89bpAsANVckv1T8I7QT6qGvyBrABut7Z8t3oEE5r1yX
UPGcOtkoRniM1h276ex9VtoGr09sUn7duoLiEsp8aip7p7SB3X6XXWJ9K733co6C
dpXotfO0zMnv8l3O9h4pHrrBkmWDBEKbUeuE9Zz7uy6mFAECQQDygylLjzX+2rvm
FYd5ejSaLEeK17AiuT29LNPRHWLu6a0zl923299FCyHLasFgbeuLRCW0LMCs2SKE
Y+cIWMSRAkEA7AnzWjby8j8efjvUwIWh/L5YJyWlSgYKlR0zdgKxxUy9+i1MGRkn
m81NLYza4JLvb8/qjUtvw92Zcppxb7E7wQJAIuQWC+X12c30nLzaOfMIIGpgfKxd
jhFivZX2f66frkn2fmbKIorCy7c3TIH2gn4uFmJenlaV/ghbe/q3oa7L0QJAFP19
ipRAXpKGX6tqbAR2N0emBzUt0btfzYrfPKtYq7b7XfgRQFogT5aeOmLARCBM8qCG
tzHyKnTWZH6ff9M/AQJBAIToUPachXPhDyOpDBcBliRNsowZcw4Yln8CnLqgS9H5
Ya8iBJilFm2UlcXfpUOk9bhBTbgFp+Bv6BZ2Alag7pY=
-----END RSA PRIVATE KEY-----";
if (!$privateKey = openssl_pkey_get_private($private)) die('Loading Private Key failed');
//Decrypt
$decrypted_text = "";
if (!openssl_private_decrypt(base64_decode($_POST['password']), $decrypted_text, $privateKey)) die('Failed to decrypt data');
//Decrypted :)
var_dump($decrypted_text);
//Free key
openssl_free_key($privateKey);
}
?>
Enjoy!
Solution 2
Here is an JavaScript RSA encryption library: http://www.ohdave.com/rsa/
And I think you could use something like this class to decrypt the generated encrypted string - http://www.phpclasses.org/browse/package/4121.html
Let me know if you manage get this work together, as I am myself looking into this subject (I actually found this post looking for this answer myself :P ) .
Edit: Look, I've also found this - http://www.sematopia.com/?p=275 - seems related to the previous two as well...
Solution 3
If you set up your server to use SSL then you can have encrypted transmission via ajax using https. That is probably the best way to encrypt data between javascript and php. If you want to make it yourself there is a big chance you will screw up somewhere and the system wont be secure.
Google on how to set up https for your server.
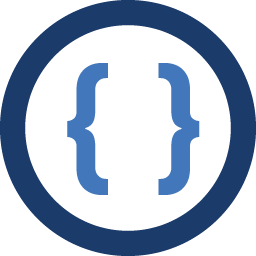
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I'd like to encrypt in Javascript and then decrypt in PHP. There are RSA implementations for Javascript and PHP but they are not compatible. I cannot decrypt in PHP correctly what I had encrypted with Javascript.
Does anyone know a library/code that will work both with Javascript and PHP?
Thanks.