Run Unix commands using PuTTY in C#
Solution 1
First, in general, you better use native .NET SSH library, like SSH.NET, instead of running external application.
See How to run commands on SSH server in C#?
-
The
putty.exe
is a GUI application. It's intended to interactive use, not for automation. There's no point trying to redirect its standard output, as it's not using it. -
For automation, use another tool from PuTTY package, the
plink.exe
.
It's a console application, so you can redirect its standard output/input. -
There's no point trying to execute an application indirectly via the
cmd.exe
. Execute it directly. -
You need to redirect standard input too, to be able to feed commands to the Plink.
-
You have to provide arguments before calling the
.Start()
. -
You may want to redirect error output too (the
RedirectStandardError
). Though note that you will need to read output and error output in parallel, what complicates the code.
static void Main(string[] args)
{
Process cmd = new Process();
cmd.StartInfo.FileName = @"C:\Program Files (x86)\PuTTY\plink.exe";
cmd.StartInfo.UseShellExecute = false;
cmd.StartInfo.RedirectStandardInput = true;
cmd.StartInfo.RedirectStandardOutput = true;
cmd.StartInfo.Arguments = "-ssh [email protected] 22 -pw mahi";
cmd.Start();
cmd.StandardInput.WriteLine("./myscript.sh");
cmd.StandardInput.WriteLine("exit");
string output = cmd.StandardOutput.ReadToEnd();
}
Solution 2
This should work:
static void Main(string[] args)
{
ProcessStartInfo cmd = new ProcessStartInfo();
cmd.FileName = @"C:\Users\win7\Desktop\putty.exe";
cmd.UseShellExecute = false;
cmd.RedirectStandardInput = false;
cmd.RedirectStandardOutput = true;
cmd.Arguments = "-ssh [email protected] 22 -pw mahi";
using (Process process = Process.Start(cmd))
{
process.WaitForExit();
}
}
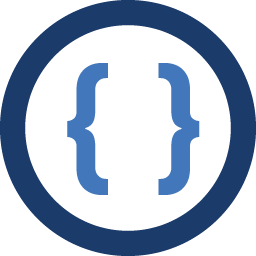
Admin
Updated on July 18, 2022Comments
-
Admin almost 2 years
I am trying to run Unix commands in PuTTY using C#. I have the below code. But the code is not working. I am not able to open PuTTY.
static void Main(string[] args) { Process cmd = new Process(); cmd.StartInfo.FileName = @"C:\Windows\System32\cmd"; cmd.StartInfo.UseShellExecute = false; cmd.StartInfo.RedirectStandardInput = false; cmd.StartInfo.RedirectStandardOutput = true; cmd.Start(); cmd.StartInfo.Arguments = "C:\Users\win7\Desktop\putty.exe -ssh [email protected] 22 -pw mahi"; }
-
Martin Prikryl over 9 yearsWhile the code indeed executes PuTTY, how does it allow you to execute commands?
-
Grady G Cooper over 9 yearsIf you want to run commands, I'd recommend using an SSH library like SSH.NET versus using putty.