Running shell script on flask
If you want to run matlab, just change your command to
cmd = ["matlab", "-nodisplay", "-nosplash", "-r", "run_image_alg"]
If you want to redirect the output to a file:
with open('text_output.txt', 'w') as fout:
subprocess.Popen(cmd, stdout=fout,
stderr=subprocess.PIPE,
stdin=subprocess.PIPE)
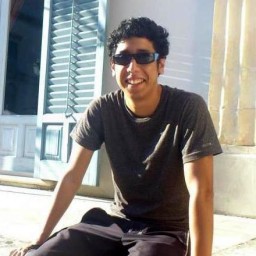
Arturo
I do research in Human Vision, but I have occasional affairs with Computer Vision.
Updated on June 04, 2022Comments
-
Arturo about 1 year
My app has been setup with the following app.py file and two .html files: index.html (base template), and upload.html where the client can see the images that he just uploaded. The problem I have is that, I want my program (presumable app.py) to execute a matlab function before the user is redirected to the upload.html template. I've found Q&A's about how to run bash shell commands on flask (yet this is not a command), but I haven't found one for scripts.
A workaround that I got was to create a shell script: hack.sh that will run the matlab code. In my terminal this is straight forward:
$bash hack.sh
hack.sh:
nohup matlab -nodisplay -nosplash -r run_image_alg > text_output.txt &
run_image_alg is my matlab file (run_image_alg.m)
Here is my code for app.py:
import os from flask import Flask, render_template, request, redirect, url_for, send_from_directory from werkzeug import secure_filename # Initialize the Flask application app = Flask(__name__) # This will be th path to the upload directory app.config['UPLOAD_FOLDER'] = 'uploads/' # These are the extension that we are accepting to be uploaded app.config['ALLOWED_EXTENSIONS'] = set(['png','jpg','jpeg']) # For a given file, return whether it's an allowed type or not def allowed_file(filename): return '.' in filename and \ filename.rsplit('.',1)[1] in app.config['ALLOWED_EXTENSIONS'] # This route will show a form to perform an AJAX request # jQuery is loaded to execute the request and update the # value of the operation @app.route('/') def index(): return render_template('index.html') #Route that will process the file upload @app.route('/upload',methods=['POST']) def upload(): uploaded_files = request.files.getlist("file[]") filenames = [] for file in uploaded_files: if file and allowed_file(file.filename): filename = secure_filename(file.filename) file.save(os.path.join(app.config['UPLOAD_FOLDER'],filename)) filenames.append(filename) print uploaded_files #RUN BASH SCRIPT HERE. return render_template('upload.html',filenames=filenames) @app.route('/uploads/<filename>') def uploaded_file(filename): return send_from_directory(app.config['UPLOAD_FOLDER'],filename) if __name__ == '__main__': app.run( host='0.0.0.0', #port=int("80"), debug=True )
I might presumably be missing a library? I found a similar Q&A on stackoverflow where someone wanted to run a (known) shell command ($ls -l). My case is different since it's not a known command, but a created script:
from flask import Flask import subprocess app = Flask(__name__) @app.route("/") def hello(): cmd = ["ls","-l"] p = subprocess.Popen(cmd, stdout = subprocess.PIPE, stderr=subprocess.PIPE, stdin=subprocess.PIPE) out,err = p.communicate() return out if __name__ == "__main__" : app.run()