RxSwift How to subscribe a observable only one time?
Solution 1
The simplest solution for what you are looking for is indeed the method that they provided for just that - func take(_ count: Int)
.
Here is a playground sample:
import RxSwift
var variable = Variable(1)
variable.asObservable().take(1).subscribe(
onNext: { print("onNext: \($0)") },
onError: { print("onError: \($0)") },
onCompleted: { print("onCompleted") },
onDisposed: { print("onDisposed") })
variable.value = 2
print("done")
The results are:
onNext: 1
onCompleted
onDisposed
done
And yes, this is useful in places where you want to filter an event through a stream, in a location where it is awkward to store a subscription.
Solution 2
Why do you want to do that? What problem are you specifically trying to solve?
The usual way to dispose subscriptions is to use a dispose bag.
func subscribeMyObservable()
{
disposeBag = DisposeBag()
myObservable
.subscribe(onNext: { print("Whatever") }
.addDisposableTo(disposeBag)
}
Notice how I recreate the disposable every time before subscribing? That would get rid of the previous subscriptions.
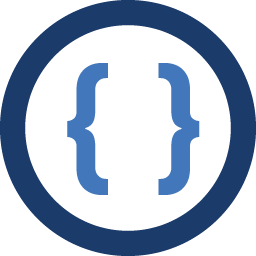
Admin
Updated on July 26, 2022Comments
-
Admin almost 2 years
I want to subscribe to an observable, but in some logic, I will re-subscribe to it. If I don't want to write some special logic for it, how can I dispose the last subscription when I add a new one? Or, when I subscribe to it, how can I know if this observable has been subscribed to already?