Safari and Chrome doesn't evaluate dynamically added <script> tag at page load
Solution 1
Try this instead:
var s = document.createElement('SCRIPT');
s.charset = 'UTF-8';
s.src ='foo.js';
document.getElementsByTagName('HEAD')[0].appendChild(s);
But place this in a SCRIPT tag at the beginning of the BODY tag
Solution 2
At the time when your javascript is evaluated, the DOM isn't fully built, the head element is not even finished. You normally cannot access or manipulate the DOM ( getElementsByTagName
and appendChild
, etc. ) before the HTML page is fully loaded.
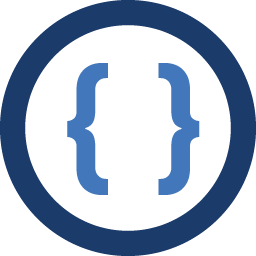
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I am writing small JavaScript code which will load external js files at html page loading.
I tested 2 ways.
Case 1: Used document.write to add <script> tag. It worked for all browsers (IE, FF, Safari, Chrome, Opera).
Case 2: Used DOMElement.appendChild to add <script> tag to the <haed> element.
Only worked for IE, FF, and Opera. Did NOT work for Safari and Chrome.
In both cases, I expected new <script> tag is being inserted before <head> closing tag. So the new <script> tag is evaluated before processing <body>, and by the time window.onload is called, "success" variable should be true.
But it wasn't for Safari and Chrome.
Can anyone tell if this should or shouldn't work? Thank you.
<html> <head> <title>JavaScript loading test</title> <script type="text/javascript"> var success = false; window.onload = function() { document.getElementById("result").innerHTML = success? "OK": "ERROR!"; } // TEST CASE 1. // Works for all browsers. document.write('<script type="text/javascript" src="foo.js"></' + 'script>'); // TEST CASE 2. // Only works for Opera, FireFox, Opera. // var scriptElem = document.createElement("script"); // scriptElem.setAttribute("type", "text/javascript"); // scriptElem.setAttribute("src", "foo.js"); // var headElem = document.getElementsByTagName("head")[0]; // headElem.appendChild(scriptElem); </script> <!-- expected new scrip tag being inserted here. --> </head> <body> Testing...<br/> <span id="result"></span> </body> </html>
"foo.js" is just one line of code.
success = true;
-
Amit Patil about 14 yearsYou can access the DOM before the page is loaded, but indeed you can't access elements below the current script block, and it's highly unreliable to do manipulations like inserts inside elements whose close-tag has not yet been encountered.