Save current page as HTML to server
Solution 1
If you meant saving the output of a page in a file, you can use buffering to do that. The function you need to use are ob_start and ob_get_contents.
<?php
// Start the buffering //
ob_start();
?>
Your page content bla bla bla bla ...
<?php
echo '1';
// Get the content that is in the buffer and put it in your file //
file_put_contents('yourpage.html', ob_get_contents());
?>
This will save the content of the page in the file yourpage.html
.
Solution 2
I think we can use Output Control Functions of PHP, you can use save the content to the variable first and then save them to the new file, next time, you can test it the html file exists, then render that else re-generate the page.
<?php
$cacheFile = 'cache.html';
if ( (file_exists($cacheFile)) && ((fileatime($cacheFile) + 600) > time()) )
{
$content = file_get_contents($cacheFile);
echo $content;
} else
{
ob_start();
// write content
echo '<h1>Hello world to cache</h1>';
$content = ob_get_contents();
ob_end_clean();
file_put_contents($cacheFile,$content);
echo $content;
}
?>
Example taken from : http://www.php.net/manual/en/function.ob-start.php#88212
Solution 3
Use JavaScript to send document.getElementsByTagName('html')[0].innerHTML
as hidden input value or by ajax to the server side. This is more useful than output buffering if the content is afterwards traversed/modified by JavaScript, which the server side might not have any notion about.
Solution 4
In case you are looking to save complete html page along with css, images and scripts in a single html file, you can use this class I have written:
This class can save HTML pages complete with images, CSS and JavaScript.
It takes the URL of a given page and retrieves it to store in a given file.
The class can parse the HTML and determine which images, CSS and JavaScript files it needs, so those files are also downloaded and saved inside the HTML page saved to a local file.
Optionally it can skip the JavaScript code, keep only the page content, and compress the resulting page removing the whitespace.
http://www.phpclasses.org/package/8305-PHP-Save-HTML-pages-complete-with-images-CSS-and-JS.html
Solution 5
I feel you need curl, so that you can save any pages' output. Use curl with returntransfer true. and do whatever you want with the output.
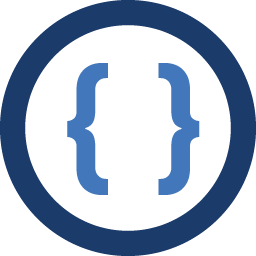
Admin
Updated on January 14, 2020Comments
-
Admin over 4 years
What approach could someone suggest to save the current page as an HTML file to the server? In this case, also note that security is not an issue.
I have spent endless hours searching around for this, and have not found a single thing.
Your help is much appreciated, thank you!
Edit
Thank you all for your help, it was very much appreciated.
-
Admin over 13 yearsThanks, BalusC. So if I use var $s = document.getElements... (in php) I can then write the whole var to a file on the server?
-
BalusC over 13 yearsJavaScript runs at webbrowser, not at webserver. Do you know JS? Anyway, given your comment I think this answer is after all not what you need :) You probably rather want to save the immediate PHP-generated HTML page, not the currently opened HTML page (in all its current client side state). Check Holyvier's answer.
-
asdfkjasdfjk over 11 yearsThank you so much. You just saved my life.
-
AlwaysANovice about 10 yearsI also had the same question. Thanks for the answer. BUT where is this
yourpage.html
being saved? I couldn't find the file in my directory folder -
HoLyVieR about 10 years@Walahh Unless
chdir
has been called, it should be in the same folder of the requested script. If you are unsure what it is, you can callgetcwd
. -
Hassan Saeed over 7 yearsi was expecting bunch of code but now i feel lucky, thanks for mini code :)
-
Woody over 5 yearsThis is a great suggestion but appears you must enter a complete URL ($txt = httpPost(<url>, "") ) instead of using a local file. maybe show us how to get a local file instead of remote URL?