save file with JFileChooser save dialog
Solution 1
JFileChooser just returns the File object, you'll have to open a FileWriter and actually write the contents to it.
E.g.
if (returnVal == JFileChooser.APPROVE_OPTION) {
File file = fc.getSelectedFile();
FileWriter fw = new FileWriter(file);
fw.write(contents);
// etc...
}
Edit:
Assuming that you simply have a source file and destination file and want to copy the contents between the two, I'd recommend using something like FileUtils from Apache's Commons IO to do the heavy lifting.
E.g.
FileUtils.copy(source, dest);
Done!
Solution 2
Just in addition to Kris' answer - I guess, you didn't read the contents of the file yet. Basically you have to do the following to copy a file with java and using JFileChooser:
- Select the source file with the FileChooser. This returns a File object, more or less a wrapper class for the file's filename
- Use a FileReader with the File to get the contents. Store it in a String or a byte array or something else
- Select the target file with the FileChooser. This again returns a File object
- Use a FileWriter with the target File to store the String or byte array from above to that file.
The File Open Dialog does not read the contents of the file into memory - it just returns an object, that represents the file.
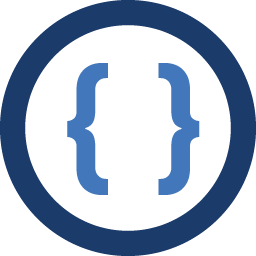
Admin
Updated on July 23, 2022Comments
-
Admin almost 2 years
I have written a Java program that opens all kind of files with a JFileChooser. Then I want to save it in another directory with the JFileChooser save dialog, but it only saves an empty file. What can I do for saving part?
Thanks.