Save HTML table as an image
Solution 1
While you can render HTML using a variety of tools, you can't be sure that those tools will render the HTML the same way your browser renders it.
If your end goal is to generate an image with text on it, then let's solve that problem instead of trying to make the solution you suggested work.
Have a look at PHP's imagettftext() function. It contains an Example #1 which creates a small, simple image from text that can be stored in any variable ... including a form variable.
By using this example, and adding a few other of PHP's GD functions, you can make a decent replica of a table, and make sure it looks exactly the way you want it, rather than the way html2ps or some other tool renders it.
<?php
// Set the content-type
header('Content-Type: image/png');
// Create the image
$im = imagecreatetruecolor(400, 30);
// Create some colors
$white = imagecolorallocate($im, 255, 255, 255);
$grey = imagecolorallocate($im, 128, 128, 128);
$black = imagecolorallocate($im, 0, 0, 0);
imagefilledrectangle($im, 0, 0, 399, 29, $white);
// The text to draw
$text = isset($_POST['name']) ? $_POST['name'] : "name";
// Replace path by your own font path
$font = 'arial.ttf';
// Add some shadow to the text
imagettftext($im, 20, 0, 11, 21, $grey, $font, $text);
// Add the text
imagettftext($im, 20, 0, 10, 20, $black, $font, $text);
// Using imagepng() results in clearer text compared with imagejpeg()
imagepng($im);
imagedestroy($im);
?>
Here's sample output generated by the above script:
Note that you'll need to provide arial.ttf
per the instructions at the link above.
If things don't work, look for errors both on-screen and in your web server's error log FIRST, before following up here. It's possible that PHP's GD module is not installed on your web server. If this is the case, you should check with your server administrator to make sure what you need is available to you.
Solution 2
You can try this JS library on client side.
Solution 3
workable solution
- use fpdf to create pdf from html (table)
- use imagemagick to convert pdf to png
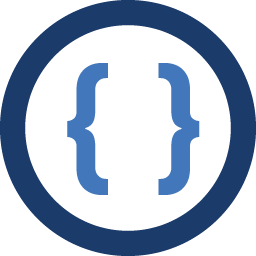
Admin
Updated on July 25, 2022Comments
-
Admin almost 2 years
I have a website I am building that takes data (the person's name) from a form and posts it into a customized "note" in a table on the next page. I want the user to then be able to save their "note" as an image with their customized name on it. Is there a way to save a SPECIFIC HTML TABLE as a PNG? Maybe take a screenshot of a specific coordinate on a web page?
If not is there a way to render posted PHP content from the form in an HTML canvas?
Any help would be great. I'm in a little over my head right now.
-
Arthur Camara almost 8 yearsTo me this is a much cleaner solution as well. JSFiddle example with the suggested library (html2canvas) here: jsfiddle.net/arthurcamara/6phhb5g9
-
Arthur Camara almost 8 yearsI think doing it server-side is an overkill for this task, which can be accomplished in a much simpler way with the library suggested by @Anber . Example here jsfiddle.net/arthurcamara/6phhb5g9
-
ghoti almost 8 years@ArthurCamara - I agree doing this client-side makes for a cleaner solution, but the OP's question was specifically about how to generate a PNG within PHP.
-
ghoti almost 8 yearsThat's a useful library. It would also be great if this answer provided context for the link, and addressed the requirement stated in the question: "I want the user to then be able to save their "note" as an image with their customized name on it."
-
Arthur Camara almost 8 yearsI think his question was about generating PNG with the table. and "If not is there a way to render posted PHP". So PHP was a 2nd option, as it should be :)
-
Arthur Camara almost 8 yearsAgreed with @ghoti :) More context and a deeper answer would be awesome. A note with their customized name on the image is also possible with canvas, so it should be no problem coming up with a client-side solution for this.