Save MultipartFileData file to disk
15,168
Here is the sample for saving file in server. Hope this will help you.
public class TestController : ApiController
{
const string StoragePath = @"T:\WebApiTest";
public async Task<HttpResponseMessage> Post()
{
if (Request.Content.IsMimeMultipartContent())
{
var streamProvider = new MultipartFormDataStreamProvider(Path.Combine(StoragePath, "Upload"));
await Request.Content.ReadAsMultipartAsync(streamProvider);
foreach (MultipartFileData fileData in streamProvider.FileData)
{
if (string.IsNullOrEmpty(fileData.Headers.ContentDisposition.FileName))
{
return Request.CreateResponse(HttpStatusCode.NotAcceptable, "This request is not properly formatted");
}
string fileName = fileData.Headers.ContentDisposition.FileName;
if (fileName.StartsWith("\"") && fileName.EndsWith("\""))
{
fileName = fileName.Trim('"');
}
if (fileName.Contains(@"/") || fileName.Contains(@"\"))
{
fileName = Path.GetFileName(fileName);
}
File.Move(fileData.LocalFileName, Path.Combine(StoragePath, fileName));
}
}
return Request.CreateResponse(HttpStatusCode.OK);
}
else
{
return Request.CreateResponse(HttpStatusCode.NotAcceptable, "This request is not properly formatted");
}
}
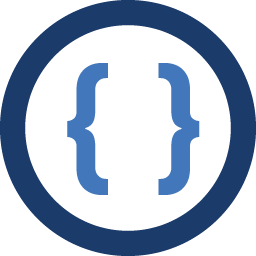
Author by
Admin
Updated on June 13, 2022Comments
-
Admin almost 2 years
I have the bellow code which I have seen in other threads here, but nowhere does it actually show how to save a MultipartFileData file to disk once you have it.
[HttpPost] public Task<HttpResponseMessage> PostFormData() { // Check if the request contains multipart/form-data. if (!Request.Content.IsMimeMultipartContent()) { throw new HttpResponseException(HttpStatusCode.UnsupportedMediaType); } string root = HttpContext.Current.Server.MapPath(Settings.AssetImageTempStorage); var provider = new MultipartFormDataStreamProvider(root); // Read the form data and return an async task. var task = Request.Content.ReadAsMultipartAsync(provider). ContinueWith<HttpResponseMessage>(t => { if (t.IsFaulted || t.IsCanceled) { Request.CreateErrorResponse(HttpStatusCode.InternalServerError, t.Exception); } // This illustrates how to get the file names. foreach (MultipartFileData file in provider.FileData) { // HOW DO I SAVE THIS FILE TO DISK HERE ??? Trace.WriteLine(file.Headers.ContentDisposition.FileName); Trace.WriteLine("Server file path: " + file.LocalFileName); } return Request.CreateResponse(HttpStatusCode.OK); }); return task; }
-
enorl76 almost 9 yearsI'm having trouble with
File.Move(fileData.LocalFileName, Path.Combine(StoragePath, fileName));
occasionally getting "File in use" exception. -
Abhilash Augustine almost 9 years@enorl76 Sorry for the late reply. I think there should not get the exception of "File in use". But, if you got this exception you can try File.Copy() instead of File.Move(). Because, method File.Copy() will be executed even the source file is in use by another process. System.IO.File.Copy(@"E:\asd.txt", @"F:\asd_copy.txt", true);
-
Shimmy Weitzhandler over 6 yearsWhere can I find how to upload the data in the client side (.NET)?
-
Abhilash Augustine over 6 years@Shimmy Sorry, I didn't get your question?
-
Shimmy Weitzhandler over 6 years@AbhilashPA-Pullelil 1. Your code shows the server side code, I was rather looking for the server side code. 2. Is the file uploaded via streaming?
-
Shimmy Weitzhandler over 6 yearsYep. I'm doubting between the variety of the options, which of them is the fastest... I can send
ByteArrayContent
,StreamContent
,PushStreamContent
, or inside a multipart with an attachment disposition. -
Abhilash Augustine over 6 years@Shimmy Oh, sorry. I am not in a position to answer this as I didn't tried any other types. If you can experiment it and share your valuable conclusions with us, it will be very helpful for all.