saving and loading objects from file using jsonpickle
21,501
Make sure that use_jsonpickle == True
in json_load_file()
. It seems that you serialize using jsonpickle
and load using json
.
>>> import jsonpickle
>>> class A(object):
... def __init__(self, name):
... self.name = name
...
>>> js = jsonpickle.encode(A('abc'))
>>> js
'{"py/object": "__main__.A", "name": "abc"}' # <-- json string
>>> a = jsonpickle.decode(js)
>>> a
<__main__.A object at 0x7f826a87bd90> # <-- python object
>>> a.name
u'abc'
>>> import json
>>> b = json.loads(js)
>>> b
{u'py/object': u'__main__.A', u'name': u'abc'} # <-- dictionary
Make sure that object type is available
>>> del A
>>> c = jsonpickle.decode(js) # no type available
>>> c
{u'py/object': u'__main__.A', u'name': u'abc'}
>>> type(c)
<type 'dict'>
>>> class A(object):
... def __init__(self, name):
... self.name = name
...
>>> d = jsonpickle.decode(js) # type is available
>>> d
<__main__.A object at 0x7f826a87bdd0>
>>> type(d)
<class '__main__.A'>
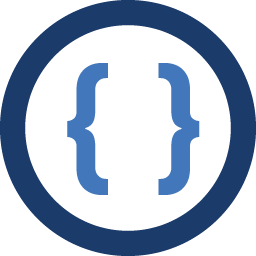
Author by
Admin
Updated on July 02, 2020Comments
-
Admin almost 4 years
I have the following simple methods for writing a python object to a file using jsonpickle:
def json_serialize(obj, filename, use_jsonpickle=True): f = open(filename, 'w') if use_jsonpickle: import jsonpickle json_obj = jsonpickle.encode(obj) f.write(json_obj) else: simplejson.dump(obj, f) f.close() def json_load_file(filename, use_jsonpickle=True): f = open(filename) if use_jsonpickle: import jsonpickle json_str = f.read() obj = jsonpickle.decode(json_str) else: obj = simplejson.load(f) return obj
the problem is that whenever I use these, it loads my objects back as dictionaries (that have fields like: "py/object": "my_module.MyClassName") but not as an actual Python object of the type that was used to generate the json string. How can I make it so jsonpickle actually converts the loaded string back to the object?
to illustrate this with an example, consider the following:
class Foo: def __init__(self, hello): self.hello = hello # make a Foo obj obj = Foo("hello world") obj_str = jsonpickle.encode(obj) restored_obj = jsonpickle.decode(obj_str) list_objects = [restored_obj] # We now get a list with a dictionary, rather than # a list containing a Foo object print "list_objects: ", list_objects
This yields:
list_objects: [{'py/object': 'as_events.Foo', 'hello': 'hello world'}]
Rather than something like: [Foo()]. How can I fix this?
thanks.
-
ed22 over 7 yearsCan anyone tell me what is this "u" thing in {u'py/object': u'main.A', u'name': u'abc'} ?
-
jfs over 7 years@ed22 it is how unicode string literals are written in Python 2.
repr(unicode_string)
uses the same text representation. -
ed22 over 7 yearsThank you so much!