Scala: abstract type pattern A is unchecked since it is eliminated by erasure
12,981
Solution 1
You could use ClassTag
like in this answer.
But I'd prefer this approach:
def myFunc(recover: PartialFunction[Throwable, Unit]): Unit = {
try {
println("Hello world") // or something else
} catch {
recover
}
}
Usage:
myFunc{ case _: MyException => }
Using ClassTag
:
import scala.reflect.{ClassTag, classTag}
def myFunc[A <: Exception: ClassTag](): Unit = {
try {
println("Hello world") // or something else
} catch {
case a if classTag[A].runtimeClass.isInstance(a) =>
}
}
Note also that in general you should use Try
with recover
method: Try
will catch only NonFatal
exceptions.
def myFunc(recover: PartialFunction[Throwable, Unit]) = {
Try {
println("Hello world") // or something else
} recover {
recover
}.get // you could drop .get here to return `Try[Unit]`
}
Solution 2
For every type check (e.g. case a: A
) the JVM needs the corresponding class
object to perform the check. In your case the JVM does not have the class object, because A
is a variable type parameter. However, you can add additional information about A
by implicitly passing a Manifest[A]
to myFunc
. As a shorthand you can just add : Manifest
to your type declaration of A
:
def myFunc[A <: Exception : Manifest]() {
try {
println("Hello world") // or something else
} catch {
case a: A => // warning: abstract type pattern A is unchecked since it is eliminated by erasure
}
}
Related videos on Youtube
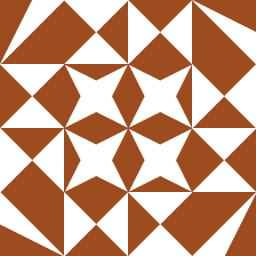
Author by
tmporaries
Updated on August 31, 2022Comments
-
tmporaries 4 months
I am writing the function that can catch exceptions of the certain type only.
def myFunc[A <: Exception]() { try { println("Hello world") // or something else } catch { case a: A => // warning: abstract type pattern A is unchecked since it is eliminated by erasure } }
What is the corrent way to bypass jvm type erasure in such case?