scala: yield pairwise combinations of two loops
11,801
Solution 1
You were almost there:
scala> val xs = List (1,2,3)
xs: List[Int] = List(1, 2, 3)
scala> val ys = List (4,5,6)
ys: List[Int] = List(4, 5, 6)
scala> for (x <- xs; y <- ys) yield (x,y)
res3: List[(Int, Int)] = List((1,4), (1,5), (1,6), (2,4), (2,5), (2,6), (3,4), (3,5), (3,6))
Solution 2
A little bit more explicit according to Nicolas:
In Scala you can use multiple generators in a single for-comprehension.
val xs = List(1,2,3)
val ys = List(4,5)
for {
x <- xs
y <- ys
} yield (x,y)
res0: List[(Int, Int)] = List((1,4), (1,5), (2,4), (2,5), (3,4), (3,5))
You can even evaluate in the comprehension.
for {
x <- xs
y <- ys
if (x + y == 6)
} yield (x,y)
res1: List[(Int, Int)] = List((1,5), (2,4))
Or make an assignment.
for {
x <- xs
y <- ys
val z = x + y
} yield (x,y,z)
res2: List[(Int,Int,Int)] = List((1,4,5), (1,5,6), (2,4,6), (2,5,7), (3,4,7), (3,5,8))
Related videos on Youtube
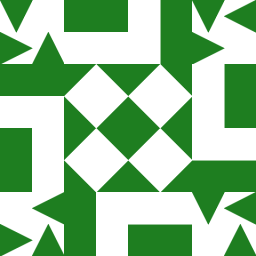
Author by
Jakub M.
Updated on September 14, 2022Comments
-
Jakub M. 3 months
I would like to create a collection with tuples containing all pairwise combinations of two lists. Something like:
for ( x <- xs ) for ( y <- ys ) yield (x,y)
In Python this would work, in Scala apparently
for
yields only for the last loop (so this evaluates toUnit
)What is the cleanest way to implement it in Scala?
-
Didier Dupont over 10 yearsNicolas's answer is the correct one, but note that
for
evaluates to Unit only when there is no yield. Checkfor(x <- xs) yield for (y <- ys) yield (x,y)
, it returns something interesting, but not exactly what you wants.
-
-
Jakub M. over 10 yearsAnd what if I would like to loop
xs
andys
and increment come countercnt
at every iteration? Like:for(x<-xs; y<-ys; cnt++) yield(x,y,cnt)
? -
tgr over 10 yearsval concatenator = for { x <- xs, y <- ys } yield (x, y); concatenator.zipWithIndex. You might flatten this result but it is counted.
-
Alex over 10 yearsfor- comprehensions are cool, i am discovering them for myself as well, and really like them especially with scalaz's validations