Screen in bash script
First, you want $test
rather than test
on the screen
lines in your script.
The -X command is expecting screen commands, not shell commands. You could look through the man page and figure out how to tell screen to type in the characters into the running shell; it's probably possible but I couldn't find it in a couple minutes. (aha, it's the screen "stuff" command: Sending input to a screen session from outside)
Alternately, you could just do something like this:
#!/bin/bash
for i in 1 2 3
do
test="test"$i
screen -dmS $test ./my_command $i
done
which will run a bare ./my_command $i in a new screen window.
You could also use a command line like this:
screen -dmS $test sh -c "ulimit 1234;./my_command $i; exec /bin/bash"
sh -c "foobar"
will cause a new shell to be run and do the foobar
command. Here we have it run several shell commands. The final exec /bin/bash
starts another shell so you don't lose the screen if ./my_command exits.
Related videos on Youtube
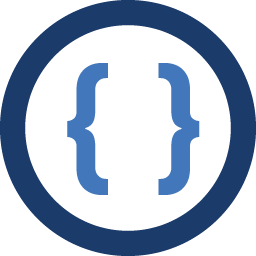
Admin
Updated on September 18, 2022Comments
-
Admin over 1 year
I am using a bash script and I need to create a screen, execute a specific command with a memory limit and detach the screen.
I have been trying something similar:
#!/bin/bash for i in 1 2 3 4 5 6 7 do test="test"$i screen -dmS test screen -r test -X ulimit -v 2199552 screen -r test -X ./my_command $i done
...but it doesn't work. In particular, the screen is created, but ./my_command is not executed. Any hint?