Searching through a text file java
40,731
package com.example;
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class FileSearch {
public void parseFile(String fileName,String searchStr) throws FileNotFoundException{
Scanner scan = new Scanner(new File(fileName));
while(scan.hasNext()){
String line = scan.nextLine().toLowerCase().toString();
if(line.contains(searchStr)){
System.out.println(line);
}
}
}
public static void main(String[] args) throws FileNotFoundException{
FileSearch fileSearch = new FileSearch();
fileSearch.parseFile("src/main/resources/test.txt", "am");
}
}
test.txt contains:
I am a legend
Hello World
I am Ironman
Output:
i am a legend
i am ironman
The above code does case insensitive search. You should use nextLine() to get the complete line. next() breaks on whitespaces.
Reference: http://docs.oracle.com/javase/7/docs/api/java/util/Scanner.html#next()
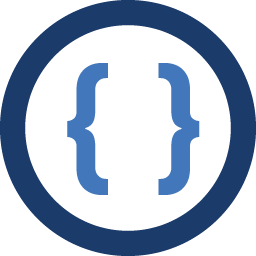
Author by
Admin
Updated on May 22, 2020Comments
-
Admin almost 4 years
So I am trying to search through a text file and if the user input is found, it returns the entire sentence including white spaces.But apparently I only get the first string and nothing pass the first string in the sentence. For example if i have a text file called "data.txt" and the contents in the first line is " I am a legend". after user enters "I am a legend" the output after the file is searched is "I". Any help would be appreciated.
public static void Findstr() { // This function searches the text for the string File file = new File("data.txt"); Scanner kb = new Scanner(System.in); System.out.println(" enter the content you looking for"); String name = kb.next(); Scanner scanner; try { scanner = new Scanner(file).useDelimiter( ","); while (scanner.hasNext()) { final String lineFromFile = scanner.nextLine(); if (lineFromFile.contains(name)) { // a match! System.out.println("I found " + name); break; } } } catch (IOException e) { System.out.println(" cannot write to file " + file.toString()); }