select xml child element with jQuery
Solution 1
Maybe something like this:
var xmlString = "<route><name>Lakeway</name><abrv>LAKE</abrv><eta><time>00:30</time><dest>MAIN</dest></eta></route>",
xmlDoc = $.parseXML( xmlString ),
$xml = $( xmlDoc ),
$abrv = $xml.find( "abrv" ).text() == "LAKE" ? $xml.find( "abrv" ) : false;
var time = $abrv.next().children("time").text();
var dest = $abrv.next().children("dest").text();
alert(time + " " + dest);
http://jsfiddle.net/jensbits/UH2m5/
Hopefully, that could get you started.
Solution 2
You could use the contains
filter to filter nodes based on this value.
Something like,
$(xml).find('abrv:contains(LAKE) + eta > *')
will return a jQuery object with two elements for the sample document.
<time>00:30</time>
<dest>MAIN</dest>
Here's an example.
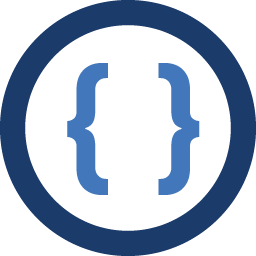
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
Is it possible to find an xml element by searching with the value of a child element?
So for XML such as:
<route> <name>Lakeway</name> <abrv>LAKE</abrv> <eta> <time>00:30</time> <dest>MAIN</dest> </eta> </route>
If I only have the value of the abrv, how would I parse the xml so as to get at the eta tags' child elements?
Is this doable with jQuery without looping through each element and comparing the values to the defined variable? Or is there a better way to do this?
EDIT:
I am trying to parse an XML feed from another domain. I'm not sure if I can do that with the xmlParse function, or if I'll need to use jQuery's Ajax functions?
-
Admin over 12 yearsHow could I use this with an external XML file? That's what I was looking for actually.
-
jk. over 12 yearsYou will need to bring the xml in using another method. See stackoverflow.com/questions/1292486/…
-
Admin over 12 yearsOK, so I used a simple proxy to get the XML, but I have another question. Is there a difference between using the parseXML function and using the $.get ajax function? So if instead of declaring an xml string, if I use $.get to get xml, should I be able to use the same selectors as you outline above?
-
jk. over 12 yearsYes, you should be able to on the return xml.