Selenium in Python on Mac - Geckodriver executable needs to be in PATH
Solution 1
Download the geckodriver and put it in /usr/local/bin; then use webdriver.Firefox like this:
from selenium import webdriver
driver = webdriver.Firefox(executable_path = '/usr/local/bin/geckodriver')
Solution 2
Perhaps someone can explain why the path isn't found. And I also hope this helps someone else troubleshoot their own path issues.
You can certainly put the geckodriver executible anywhere you'd like. On my Mac, I chose ~/.local/bin since its a common place for executables to be stored that are specific to a user account. For example. the Heroku CLI is placed in ~/.local/share. This approach also eliminates the need for superuser access when adding an executable to a system location like /usr/local/bin
I then added it to the path within my .profile with
EXPORT PATH=$PATH:~/.local/bin
I tested by opening a terminal and checking with:
geckodriver --version
which worked fine.
But from a Python virtual environment, for some reason, the system path isn't passed?? I discovered this by adding to my selenium test script:
import sys
for p in sys.path:
print(p)
Which showed:
/Users/philip/Devel/myproject
/Users/philip/.virtualenvs/myproject/lib/python36.zip
/Users/philip/.virtualenvs/myproject/lib/python3.6
/Users/philip/.virtualenvs/myproject/lib/python3.6/lib-dynload
/Library/Frameworks/Python.framework/Versions/3.6/lib/python3.6
/Users/philip/.virtualenvs/myproject/lib/python3.6/site-packages
So ultimately I had to specify the path with:
self.browser = webdriver.Firefox(executable_path=r'/Users/philip/.local/bin/geckodriver')
This approach works fine, but I'd still like to know why I couldn't set the path in the virtual environment.
Solution 3
If you're on MACos and you're running PyCharm, I too couldn't get my project to find anything in /usr/local/bin, where I had geckodriver installed. $PATH was correct and I was able to run it in Terminal on any folder. So I copied the binary over to \venv\bin for the project I am running and it worked like a charm - PyCharm in fact! :P
Solution 4
simply download the executable that matches your os from here executables
unzip and put the executable in desired folder in your project
use **os ** or any path library to get the path your executable
import os
from selenium import webdriver
path_executable = os.path.abs( path/to/executable )
browser = webdriver.Firefox( executable_path= path_executable )
or you need something flexible which will work no matter the os and no need to download executable but its slower use pip to install webdriver manager webdriver manager doc$ pip install webdriver_manager
then from selenium import webdriver
from webdriver_manager.firefox import GeckoDriverManager
browser=webdriver.Firefox(executable_path=GeckoDriverManager().install())
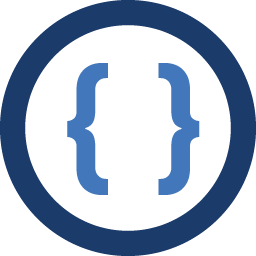
Admin
Updated on January 11, 2022Comments
-
Admin over 2 years
I'm new to programming and started with Python about 2 months ago and am going over Sweigart's Automate the Boring Stuff with Python text. I'm using Spyder 3 and already installed the selenium module and the Firefox browser. I used the following code in python file
from selenium import webdriver browser = webdriver.Firefox() browser.get('http://inventwithpython.com')
I get this error:
Message: 'geckodriver' executable needs to be in PATH.
I've downloaded geckodriver.exe in addition to going into terminal and installing it using
brew install geckodriver
Oddly enough, if I go into terminal and type "python" and then put the code in, it works, but not when I run the file in Spyder. Where do I need to put the geckodriver.exe file for it to work? I've tried putting it in various folders (same folder as the python file, same folder as the webdriver file, in the user bin, and so on) but I get the same error
I've looked at similar questions but can't seem to find something that works. I've also tried with Chrome but I get the same error but with chromedriver.
which geckodriver
yields
/usr/local/bin/geckodriver
I'm also on a Mac, so file paths are a little more difficult for me than on windows.
-
help-info.de over 3 yearsWelcome to Stack Overflow! Please note you are answering a very old and already answered question. Here is a guide on How to Answer.
-
Aashiq almost 3 yearsi installed using brew, its in , /opt/homebrew/bin/geckodriver path , what should i do?