Selenium - MoveTargetOutOfBoundsException with Firefox
Solution 1
This error...
selenium.common.exceptions.MoveTargetOutOfBoundsException: Message: (134.96666717529297, 8682.183013916016) is out of bounds of Viewport width (1268) and height (854)
...implies that the element you are looking for is not within the Viewport. We need to scroll down to bring the element within the Viewport. Here is the working code:
from selenium import webdriver
from selenium.webdriver.firefox.firefox_binary import FirefoxBinary
from selenium.webdriver.common.desired_capabilities import DesiredCapabilities
from selenium.webdriver.common.action_chains import ActionChains
binary = FirefoxBinary('C:\\Program Files\\Mozilla Firefox\\firefox.exe')
caps = DesiredCapabilities().FIREFOX
caps["marionette"] = True
driver = webdriver.Firefox(capabilities=caps, firefox_binary=binary, executable_path="C:\\Utility\\BrowserDrivers\\geckodriver.exe")
driver.get("https://stackoverflow.com")
last_height = driver.execute_script("return document.body.scrollHeight")
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
source_element = driver.find_element_by_xpath('//*[@id="footer"]/div/ul/li[1]/a')
ActionChains(driver).move_to_element(source_element).perform()
Let me know if this Answers your Question.
Solution 2
I think the correct answer here got lucky that the element they were looking for happened to be at the bottom of the page and didn't really explain why this occurs in Firefox commonly.
Browsers other than Firefox treat Webdrivers move_to_element
action as scroll to part of page with element then hover over it. Firefox seems to have taken a hardline stance that move_to_element is just hover and are waiting for a scroll action to fix this.
For now you have to workaround this bug using javascript as mentioned in previous answer, but I suggest using something like this instead of arbitrarily (well I guess the example was a footer) scrolling to bottom of page and hoping object is still in view.
def scroll_shim(passed_in_driver, object):
x = object.location['x']
y = object.location['y']
scroll_by_coord = 'window.scrollTo(%s,%s);' % (
x,
y
)
scroll_nav_out_of_way = 'window.scrollBy(0, -120);'
passed_in_driver.execute_script(scroll_by_coord)
passed_in_driver.execute_script(scroll_nav_out_of_way)
Then later
source_element = driver.find_element_by_xpath('//*[@id="footer"]/div/ul/li[1]/a')
if 'firefox' in driver.capabilities['browserName']:
scroll_shim(driver, source_element)
# scroll_shim is just scrolling it into view, you still need to hover over it to click using an action chain.
actions = ActionChains(driver)
actions.move_to_element(source_element)
actions.click()
actions.perform()
Solution 3
You can try below while automating the script in Firefox when it usually throws MoveTargetOutOfBoundsException error:
One can transform/Zoom-in or out by
driver.execute_script("document.body.style.transform='scale(0.9)';")
Sometimes if you are running automation script in Jenkins(CI tools), you might also face the issue from above transform code where content of browser is scaled out not the actual browser, in those condition you can try out to resize the browser window:
driver.set_window_size(x, y)
or
driver.set_window_size(2000, 694)
Related videos on Youtube
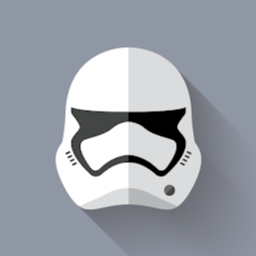
Matheus
Updated on July 09, 2022Comments
-
Matheus 6 months
I'v got problem with function move_to_element on Firefox Webdriver (Chrome, IE works well)
driver = webdriver.Firefox() driver.get("https://stackoverflow.com") time.sleep(5) source_element = driver.find_element_by_xpath('//*[@id="footer"]/div/ul/li[1]/a') ActionChains(driver).move_to_element(source_element).perform()
I am working with these versions: geckodriver - 0.17.0 // Firefox - 54.0 // selenium - 3.4.3
After running this script, on output shows:
selenium.common.exceptions.MoveTargetOutOfBoundsException: Message: (134.96666717529297, 8682.183013916016) is out of bounds of viewport width (1268) and height (854)
-
Cynic about 4 yearsOh man, they might finally fix this: w3.org/2018/10/26-webdriver-minutes.html#resolution01
-
Eliezer Miron about 4 yearsI tried using your function, but it isn't scrolling at all. I then get the expected MoveTargetOutOfBoundsException when I try to access the element. Do I need to change anything for Python 3?
-
Cynic about 4 yearsYou need to pass in the element and driver but it should just work. What browser are you using? Also try doing something like
import time
andtime.sleep(3)
after you call it so you can see it scroll -
Eliezer Miron about 4 yearsI'm using Firefox. I'll try the sleeps. EDIT: Sleeps didn't help. I'm passing it the driver and element, and I know the function is running, it just doesn't scroll at all. I'm in a unique position where only part of the frame is scrollable, so I'm clicking in that area before trying this function. I guess that part may be failing.
-
Eliezer Miron about 4 yearsI ended up using <driver.execute_script("arguments[0].scrollIntoView();", element)> and it actually worked fine. Not sure why that worked but your function didn't.
-
Cynic about 4 yearsYeah, the exception is happening when you click. So if you don't use the function to scroll to it before you click you would get the exception. The function works fine in Firefox. Asked about browser as I haven't tested it IE but it's basic javascript so I would expect it should work.
-
Eliezer Miron about 4 yearsI thought your scroll_shim function was supposed to the scrolling?
-
Cynic about 4 yearsIt does but you have to scroll before you click. So it wasn't working because you were hitting the error before you were calling the function. I am using this with Firefox and it works.
-
Eliezer Miron about 4 yearsI had a time.sleep between scroll_shim and the click call. It's probably just related to the weirdness of the HTML I was working with, so I wouldn't worry about it.
-
ishandutta2007 over 3 yearswhat is
last_height
for ? -
Erin B over 3 years@Cynic I see that w3 has updated some documents on this here: drafts.csswg.org/cssom-view/#dom-element-scrollintoview Do you know if there is any implementation of this yet? I've got a bunch of code for testing in Chrome that I was trying to use for FireFox, and I don't want to rewrite the existing code if I can help it.
-
Cynic over 3 yearsEliezer Miron in a previous comment mentioned how you could use
driver.execute_script("arguments[0].scrollIntoView();
instead. But as far as them fixing it in selenium, I doubt anything would happen before Selenium 4, even with that, it doesn't sound like Firefox would makemove_to_element
consistent. We'd probably just have to add something likescroll_to_element
to all our action chains to support all browsers. So the fix would likely be similar to creating your own scroll shim and pasting it in before your clicks. -
Mate Ajdukovic over 2 yearsThat's just bad solution.
-
Nitin over 2 yearsAdding a simple element.click() before the actions, worked for me :)
-
m3nda almost 2 yearsShould be wrapped with WebDriverWait.