Send a JSON Object from Android to PHP server with POST method and HttpURLConnection
26,593
Solution 1
Finally, I success to send a JSONObject to my server.
I used this code for the android part :
new Thread(new Runnable() {
@Override
public void run() {
OutputStream os = null;
InputStream is = null;
HttpURLConnection conn = null;
try {
//constants
URL url = new URL("http://192.168.43.64/happiness_barometer/php_input.php");
JSONObject jsonObject = new JSONObject();
jsonObject.put("rate", "1");
jsonObject.put("comment", "OK");
jsonObject.put("category", "pro");
jsonObject.put("day", "19");
jsonObject.put("month", "8");
jsonObject.put("year", "2015");
jsonObject.put("hour", "16");
jsonObject.put("minute", "41");
jsonObject.put("day_of_week", "3");
jsonObject.put("week", "34");
jsonObject.put("rate_number", "1");
String message = jsonObject.toString();
conn = (HttpURLConnection) url.openConnection();
conn.setReadTimeout( 10000 /*milliseconds*/ );
conn.setConnectTimeout( 15000 /* milliseconds */ );
conn.setRequestMethod("POST");
conn.setDoInput(true);
conn.setDoOutput(true);
conn.setFixedLengthStreamingMode(message.getBytes().length);
//make some HTTP header nicety
conn.setRequestProperty("Content-Type", "application/json;charset=utf-8");
conn.setRequestProperty("X-Requested-With", "XMLHttpRequest");
//open
conn.connect();
//setup send
os = new BufferedOutputStream(conn.getOutputStream());
os.write(message.getBytes());
//clean up
os.flush();
//do somehting with response
is = conn.getInputStream();
//String contentAsString = readIt(is,len);
} catch (IOException e) {
e.printStackTrace();
} catch (JSONException e) {
e.printStackTrace();
} finally {
//clean up
try {
os.close();
is.close();
} catch (IOException e) {
e.printStackTrace();
}
conn.disconnect();
}
}
}).start();
and this one in the php part :
$json = file_get_contents('php://input');
$obj = json_decode($json);
$rate = $obj->{'rate'};
$comment = $obj->{'comment'};
$category = $obj->{'category'};
$day = $obj->{'day'};
$month = $obj->{'month'};
$year = $obj->{'year'};
$hour = $obj->{'hour'};
$minute = $obj->{'minute'};
$day_of_week = $obj->{'day_of_week'};
$week = $obj->{'week'};
$rate_number = $obj->{'rate_number'};
try
{
$bdd = new PDO('mysql:host=localhost;dbname=happiness_barometer;charset=utf8', 'utilisateur', '');
} catch (Exception $e)
{
die('Erreur : '.$e->getMessage());
}
$sql = $bdd->prepare(
'INSERT INTO rates (rate, comment, category, day, month, year, hour, minute, day_of_week, week, rate_number)
VALUES (:rate, :comment, :category, :day, :month, :year, :hour, :minute, :day_of_week, :week, :rate_number)');
if (!empty($rate)) {
$sql->execute(array(
'rate' => $rate,
'comment' => $comment,
'category' => $category,
'day' => $day,
'month' => $month,
'year' => $year,
'hour' => $hour,
'minute' => $minute,
'day_of_week' => $day_of_week,
'week' => $week,
'rate_number' => $rate_number));
}
Hope it will help someone else ;)
Solution 2
Check with this code:
public class UniversalNetworkConnection {
public static String postJSONObject(String myurl, JSONObject parameters) {
HttpURLConnection conn = null;
try {
StringBuffer response = null;
URL url = new URL(myurl);
conn = (HttpURLConnection) url.openConnection();
conn.setReadTimeout(10000);
conn.setConnectTimeout(15000);
conn.setRequestProperty("Content-Type", "application/json");
conn.setDoOutput(true);
conn.setRequestMethod("POST");
OutputStream out = new BufferedOutputStream(conn.getOutputStream());
BufferedWriter writer = new BufferedWriter(new OutputStreamWriter(out, "UTF-8"));
writer.write(parameters.toString());
writer.close();
out.close();
int responseCode = conn.getResponseCode();
System.out.println("responseCode" + responseCode);
switch (responseCode) {
case 200:
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String inputLine;
response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
return response.toString();
}
} catch (IOException ex) {
ex.printStackTrace();
} finally {
if (conn != null) {
try {
conn.disconnect();
} catch (Exception ex) {
ex.printStackTrace();
}
}
}
return null;
}
}
on PHP Side:
<?php
$json = file_get_contents('php://input');
$obj = json_decode($json);
print_r($obj);
print_r("this is a test");
?>
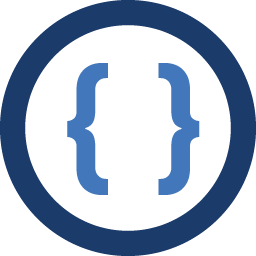
Author by
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I'm trying to establish communication between my Android app and my WampServer on local network.
When I want to read data from the server, I have had success, but I have a problem when I try to send data to the server.
I'm using an Service to established the communication :
public class SynchronisationService extends Service { @Override public IBinder onBind(Intent intent) { return null; } @Override public int onStartCommand(Intent intent, int flags, int startId) { super.onStartCommand(intent, flags, startId); new Thread(new Runnable() { @Override public void run() { try { URL url = new URL("http://192.168.37.23/happiness_barometer/php_input.php"); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); connection.setDoOutput(true); connection.setDoInput(false); connection.setRequestMethod("POST"); connection.connect(); OutputStream outputStream = connection.getOutputStream(); OutputStreamWriter writer = new OutputStreamWriter(outputStream); JSONObject jsonObject = new JSONObject(); jsonObject.put("rate", 1); writer.write(URLEncoder.encode(jsonObject.toString(), "UTF-8")); writer.flush(); writer.close(); } catch (Exception e) { Log.v("EXCEPTION", e.getMessage()); } } }).start(); stopSelf(); return flags; }
}
And my php file:
<?php try { $bdd = new PDO('mysql:host=localhost;dbname=happiness_barometer;charset=utf8', 'utilisateur', ''); } catch (Exception $e) { die('Erreur : '.$e->getMessage()); } $sql = $bdd->prepare( 'INSERT INTO rates (rate, comment, category, day, month, year, hour, minute, day_of_week, week, rate_number) VALUES (:rate, :comment, :category, :day, :month, :year, :hour, :minute, :day_of_week, :week, :rate_number)'); if (!empty($_POST['rate'])) { $sql->execute(array( 'rate' => $_POST['rate'], 'comment' => '', 'category' => 'pro', 'day' => 19, 'month' => 8, 'year' => 2015, 'hour' => 18, 'minute' => 3, 'day_of_week' =>3, 'week' => 33, 'rate_number' => 2)); } ?>
When I run my app, nothing is added to my database. I think there is nothing in the
$_POST['rate']
.Please, tell me what is wrong in my code?
-
Admin over 8 yearsI try this code but nothing happened too : no exception, no errors, nothing about System.out in logcat.
-
Bonatti over 8 years@Anaïs Check on wamp -> apache -> access log. Check if the connection was stablished
-
Admin over 8 years@Bonatti I Check the access log, the php_output file is "open" by a client on the local network (ip address : 192.168.36.212) but the php_input file is never "open". I also find a 408 error I think. Is it helpful?
-
Bonatti over 8 years@Anaïs The 408 are likely that the server did not received the full request in the time it was expecting one. Please, use cURL or some other method to perform the request from a different source, this can be used to rule out any failures in the server side. IF you had no errors from the server, then you are missing request parts on your Android device... namely at least
Content-Type
,Content-Length
andcharset
-
Skynet over 6 years@Harsha that sounds like outsourcing to me!
-
Yirga over 6 years@Anaïs i am using the same code the response i get httpUrlConnection.getResponse() is HttpURLConnection.HTTP_OK but the php is not accepting the json data. Please help
-
Yirga over 6 years@Skynet i don't have error and also i checked the access log connection was established with 200 response from the server but he php script is not reading the json data.
-
Pavel almost 6 yearsyou declared os as OutputStream, but assign it BufferedOutputStream. Not sure what API you were using, but it's not gonna work in current versions.
-
Ali Hosein pour over 4 yearsHi. I try your code. But nothing show. Please help me. Is there any problem in .php file?