send files(images) from android device to webservice on the server written using REST
20,149
Solution 1
Check the link . it gives complete example of how to upload file to server.
or check below code -
public class HttpFileUpload implements Runnable{
URL connectURL;
String responseString;
String Title;
String Description;
byte[ ] dataToServer;
FileInputStream fileInputStream = null;
HttpFileUpload(String urlString, String vTitle, String vDesc){
try{
connectURL = new URL(urlString);
Title= vTitle;
Description = vDesc;
}catch(Exception ex){
Log.i("HttpFileUpload","URL Malformatted");
}
}
void Send_Now(FileInputStream fStream){
fileInputStream = fStream;
Sending();
}
void Sending(){
String iFileName = "ovicam_temp_vid.mp4";
String lineEnd = "\r\n";
String twoHyphens = "--";
String boundary = "*****";
String Tag="fSnd";
try
{
Log.e(Tag,"Starting Http File Sending to URL");
// Open a HTTP connection to the URL
HttpURLConnection conn = (HttpURLConnection)connectURL.openConnection();
// Allow Inputs
conn.setDoInput(true);
// Allow Outputs
conn.setDoOutput(true);
// Don't use a cached copy.
conn.setUseCaches(false);
// Use a post method.
conn.setRequestMethod("POST");
conn.setRequestProperty("Connection", "Keep-Alive");
conn.setRequestProperty("Content-Type", "multipart/form-data;boundary="+boundary);
DataOutputStream dos = new DataOutputStream(conn.getOutputStream());
dos.writeBytes(twoHyphens + boundary + lineEnd);
dos.writeBytes("Content-Disposition: form-data; name=\"title\""+ lineEnd);
dos.writeBytes(lineEnd);
dos.writeBytes(Title);
dos.writeBytes(lineEnd);
dos.writeBytes(twoHyphens + boundary + lineEnd);
dos.writeBytes("Content-Disposition: form-data; name=\"description\""+ lineEnd);
dos.writeBytes(lineEnd);
dos.writeBytes(Description);
dos.writeBytes(lineEnd);
dos.writeBytes(twoHyphens + boundary + lineEnd);
dos.writeBytes("Content-Disposition: form-data; name=\"uploadedfile\";filename=\"" + iFileName +"\"" + lineEnd);
dos.writeBytes(lineEnd);
Log.e(Tag,"Headers are written");
// create a buffer of maximum size
int bytesAvailable = fileInputStream.available();
int maxBufferSize = 1024;
int bufferSize = Math.min(bytesAvailable, maxBufferSize);
byte[ ] buffer = new byte[bufferSize];
// read file and write it into form...
int bytesRead = fileInputStream.read(buffer, 0, bufferSize);
while (bytesRead > 0)
{
dos.write(buffer, 0, bufferSize);
bytesAvailable = fileInputStream.available();
bufferSize = Math.min(bytesAvailable,maxBufferSize);
bytesRead = fileInputStream.read(buffer, 0,bufferSize);
}
dos.writeBytes(lineEnd);
dos.writeBytes(twoHyphens + boundary + twoHyphens + lineEnd);
// close streams
fileInputStream.close();
dos.flush();
Log.e(Tag,"File Sent, Response: "+String.valueOf(conn.getResponseCode()));
InputStream is = conn.getInputStream();
// retrieve the response from server
int ch;
StringBuffer b =new StringBuffer();
while( ( ch = is.read() ) != -1 ){ b.append( (char)ch ); }
String s=b.toString();
Log.i("Response",s);
dos.close();
}
catch (MalformedURLException ex)
{
Log.e(Tag, "URL error: " + ex.getMessage(), ex);
}
catch (IOException ioe)
{
Log.e(Tag, "IO error: " + ioe.getMessage(), ioe);
}
}
@Override
public void run() {
// TODO Auto-generated method stub
}
}
public void UploadFile(){
try {
// Set your file path here
FileInputStream fstrm = new FileInputStream(Environment.getExternalStorageDirectory().toString()+"/DCIM/file.mp4");
// Set your server page url (and the file title/description)
HttpFileUpload hfu = new HttpFileUpload("http://www.myurl.com/fileup.aspx", "my file title","my file description");
hfu.Send_Now(fstrm);
} catch (FileNotFoundException e) {
// Error: File not found
}
}
Solution 2
You can use below code to upload image with REST webservice
try {
HttpClient httpClient = new DefaultHttpClient();
HttpContext httpContext = new BasicHttpContext();
HttpPost httpPost = new HttpPost(
"YOUR WEB SERVICE URL");
entity = getMultipleEntityUpload();
httpPost.setEntity(entity);
HttpResponse httpResponse = httpClient.execute(httpPost,
httpContext);
HttpEntity httpEntity = httpResponse.getEntity();
InputStream is = httpEntity.getContent();
String line = "";
StringBuilder total = new StringBuilder();
BufferedReader rd = new BufferedReader(new InputStreamReader(
is));
while ((line = rd.readLine()) != null) {
total.append(line);
}
String result =total.toString();
} catch (Exception e) {
// TODO: handle exception
}
private MultipartEntity getMultipleEntityUpload() {
MultipartEntity entity = new MultipartEntity(
HttpMultipartMode.BROWSER_COMPATIBLE);
try {
ByteArrayOutputStream stream = new ByteArrayOutputStream();
//imagePic is bitmap of your image
imagePic.compress(Bitmap.CompressFormat.JPEG, 100, stream);
byte[] arrByteImage = stream.toByteArray();
try {
entity.addPart(WS_Key_Constant.KEY_IMAGE, new ByteArrayBody(
arrByteImage, ".jpg"));
} catch (Exception e) {
}
} catch (Exception e) {
// TODO: handle exception
e.printStackTrace();
}
return entity;
}
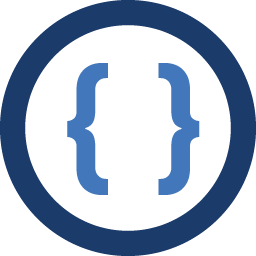
Author by
Admin
Updated on September 09, 2020Comments
-
Admin over 3 years
I want to send images from android device to my web application running on server with tomcat. please help me in writing small code for sending image to a REST web service running on Web server. Please provide me the sample code if possible. I am stuck with what method to use. Any help would be greatly appreciated. thanks in advance.
Edit: The answer for this question is as follows
while(it.hasNext()){ File file = new File((new StringBuilder()).append(Environment.getExternalStorageDirectory()).append(File.separator).append("jcms").append(File.separator).append("Customer_").append( customer.getId()).toString()); File[] listOfFiles = file.listFiles(); for(int i=0;i<listOfFiles.length;i++){ JSONObject message = new JSONObject(); File fil=listOfFiles[i]; FileInputStream imageInFile = new FileInputStream(fil); byte imageData[] = new byte[(int)fil.length()]; imageInFile.read(imageData); String imageDataString = encodeImage(imageData); URL url=new URL(ClearCustomersContract.CLEAR_SERVER_URL); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); connection.setDoOutput(true); connection.setRequestProperty("Content-Type", "application/json"); connection.setRequestMethod("POST"); connection.setConnectTimeout(5000); connection.setReadTimeout(5000); OutputStreamWriter out = new OutputStreamWriter(connection.getOutputStream()); out.write(imageDataString); out.close(); BufferedReader in = new BufferedReader(new InputStreamReader( connection.getInputStream())); while (in.readLine() != null) { } in.close(); } }
And The REST Webservice on server side is like
@Override @POST @Consumes({MediaType.APPLICATION_JSON,MediaType.APPLICATION_OCTET_STREAM}) @Path("/getData") public Response getAllTheSyncData(InputStream incomingData) { StringBuilder sb = new StringBuilder(); try { BufferedReader in = new BufferedReader(new InputStreamReader(incomingData)); String line = null; while ((line = in.readLine()) != null) { sb.append(line); } } catch (Exception e) { System.out.println("Error Parsing: - "); } return Response.status(200).entity("Success").build(); }
and this is how we convert the string back to image.
byte[] imageByteArray = decodeImage(jsonObj.get("imageData").toString());
imageOutFile = new FileOutputStream( "C:/Users/SUNILKUMAR/Desktop/result.jpg"); // Write a image byte array into file system imageOutFile.write(imageByteArray); imageOutFile.close();
-
Admin about 9 yearsHi Ravi, Thanks for the response. And also can you please give me the template for the webservice that accepts this stream on the server side.
-
Admin about 9 yearsHi Y.S. Thanks for the reply. can you please post the webservice template on the server side to accept this request.
-
Ravi Bhandari about 9 yearssorry buddy i dont have that much knowledge of webservice that i can share.