Send POST with WebClient.DownloadString in C#
24,407
Solution 1
You can use WebClient.UploadData()
which uses HTTP POST, i.e.:
using (WebClient wc = new WebClient())
{
byte[] result = wc.UploadData("http://stackoverflow.com", new byte[] { });
}
The payload data that you specify will be transmitted as the POST body of your request.
Alternatively there is WebClient.UploadValues()
to upload a name-value collection also via HTTP POST.
Solution 2
You could use Upload method with HTTP 1.0 POST
string postData = Console.ReadLine();
using (System.Net.WebClient wc = new System.Net.WebClient())
{
wc.Headers.Add("Content-Type","application/x-www-form-urlencoded");
// Upload the input string using the HTTP 1.0 POST method.
byte[] byteArray = System.Text.Encoding.ASCII.GetBytes(postData);
byte[] byteResult= wc.UploadData("http://targetwebiste","POST",byteArray);
// Decode and display the result.
Console.WriteLine("\nResult received was {0}",
Encoding.ASCII.GetString(byteResult));
}
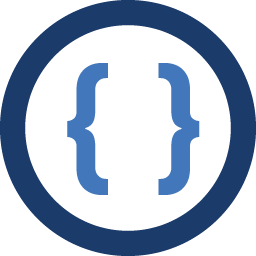
Author by
Admin
Updated on August 07, 2020Comments
-
Admin over 3 years
I know there are a lot of questions about sending HTTP POST requests with C#, but I'm looking for a method that uses
WebClient
rather thanHttpWebRequest
. Is this possible? It'd be nice because theWebClient
class is so easy to use.I know I can set the
Headers
property to have certain headers set, but I don't know if it's possible to actually do a POST fromWebClient
.