SendInput() Keyboard letters C/C++
40,617
Solution 1
INPUT input;
WORD vkey = VK_F12; // see link below
input.type = INPUT_KEYBOARD;
input.ki.wScan = MapVirtualKey(vkey, MAPVK_VK_TO_VSC);
input.ki.time = 0;
input.ki.dwExtraInfo = 0;
input.ki.wVk = vkey;
input.ki.dwFlags = 0; // there is no KEYEVENTF_KEYDOWN
SendInput(1, &input, sizeof(INPUT));
input.ki.dwFlags = KEYEVENTF_KEYUP;
SendInput(1, &input, sizeof(INPUT));
List of virtual key codes .....
Solution 2
I made a modification after reading @Nathan's code, this reference and combined with @jave.web's suggestion. This code can be used to input characters (both upper-case and lower-case).
#define WINVER 0x0500
#include<windows.h>
void pressKeyB(char mK)
{
HKL kbl = GetKeyboardLayout(0);
INPUT ip;
ip.type = INPUT_KEYBOARD;
ip.ki.time = 0;
ip.ki.dwFlags = KEYEVENTF_UNICODE;
if ((int)mK<65 || (int)mK>90) //for lowercase
{
ip.ki.wScan = 0;
ip.ki.wVk = VkKeyScanEx( mK, kbl );
}
else //for uppercase
{
ip.ki.wScan = mK;
ip.ki.wVk = 0;
}
ip.ki.dwExtraInfo = 0;
SendInput(1, &ip, sizeof(INPUT));
}
Below is the function for pressing Return key:
void pressEnter()
{
INPUT ip;
ip.type = INPUT_KEYBOARD;
ip.ki.time = 0;
ip.ki.dwFlags = KEYEVENTF_UNICODE;
ip.ki.wScan = VK_RETURN; //VK_RETURN is the code of Return key
ip.ki.wVk = 0;
ip.ki.dwExtraInfo = 0;
SendInput(1, &ip, sizeof(INPUT));
}
Solution 3
The SendInput function accepts an array of INPUT structures. The INPUT structures can either be a mouse or keyboard event. The keyboard event structure has a member called wVk which can be any key on the keyboard. The Winuser.h header file provides macro definitions (VK_*) for each key.
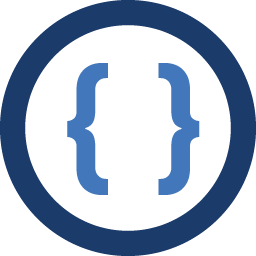
Author by
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I am trying to use
SendInput()
to send a sentence to another application (Notepad) and then send it hitting the Enter Key.Any code snippets? Or help
-
jave.web over 9 yearsUse
VkKeyScanEx( char, KeyboardLayout )
to put your "common"chars
into this example :) ...input.ki.wVk = VkKeyScanEx('a',kbl);
as for the KeyboardLayout the simpliest way is to load current window's keyboardLayout:HKL kbl = GetKeyboardLayout(0);
-
Lê Quang Duy over 7 yearsThank you and @jave.web for the answer. I made up my code for inputting character in the answer below (sorry I can't find how to insert code into comment section).
-
Talset over 4 yearsJust a note, in case others are confused: The pressEnter() function works for text fields/editors, but doesn't trigger a response in some form controls. The following post works correctly in both cases with VK_RETURN: stackoverflow.com/a/20857633/8690169
-
Bobbie E. Ray over 2 yearsLine 9 reads
if ((int)mK<65 && (int)mK>90)
, i.e. the char code, cast to an int, must be smaller than 65 and larger than 90 at the same time. I assume this must be an or, i.e.if ((int)mK<65 || (int)mK>90)
, to match special and small chars in the ASCII table, otherwise the condition is mutually exclusive and can never be met -
Lê Quang Duy over 2 yearsThank you @BobbieE.Ray for pointing this out. I edited the post following your suggestion.
-
Bobbie E. Ray over 2 yearsThanks for updating the code. If you could give my remark some kudos I'd appreciate it. I'm working my way so up that some day I can edit answers directly :) Thank you