Serial communication and CRLF C#
12,331
byte[] array = ComPort.StringToBytes(content + "\r\n");
Or use your WriteLine
method which already does this. So:
byte[] array = ComPort.StringToBytes(content);
_comport.WriteBytes(array);
Becomes:
_comport.WriteLine(content);
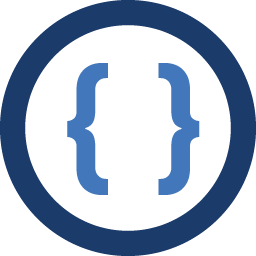
Author by
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
i Have question about CRLF after sending a string to the serial port. Let me explain what im trying to do, Under here there is an example.
[ACR] CRLF 10:90 CRLF 11:20 CRLF 12:2.1 CRLF
That is what im trying to do but i cant get anywhere, Can someone help me with this. i think i have to do the CRLF to start a newline but if you have other suggestions they will be more then welcome This is what i've done:
Code:
private void SendMessageTest() { var content = _durCleaningTextbox.Text; byte[] array = ComPort.StringToBytes(content); _comport.WriteBytes(array); _comport.ReadBytes(array, array.Length, 1000); string result = Encoding.ASCII.GetString(array); MessageBox.Show(result); }
using System; using System.IO; using System.Text; using System.IO.Ports; namespace Communication { public class ComPort { private readonly SerialPort _serialPort; public ComPort(string portName, int baudRate) { _serialPort = new SerialPort(); _serialPort.PortName = portName; _serialPort.BaudRate = baudRate; _serialPort.StopBits = StopBits.One; _serialPort.DataBits = 8; _serialPort.Parity = Parity.None; _serialPort.Handshake = Handshake.None; // _serialPort.WriteBufferSize = 1; _serialPort.DtrEnable = true; _serialPort.RtsEnable = true; _serialPort.Open(); _serialPort.ReadTimeout = 20000; _serialPort.WriteTimeout = 20000; } public void Clear() { while (ReadByte() != -1) continue; } private byte[] _array = new byte[] {0}; public void WriteByte(byte value) { _array[0] = value; _serialPort.Write(_array, 0, 1); // _serialPort.BaseStream.WriteByte(value); _serialPort.BaseStream.Flush(); } public void WriteBytes(byte[] array) { _serialPort.Write(array, 0, array.Length); } public void WriteBytes(byte[] array, int index, int length ) { _serialPort.Write(array, index, length); } private int _readTimeOut = -1; public int ReadByte(int timeOut = 200) { if (timeOut != _readTimeOut) _serialPort.ReadTimeout = _readTimeOut = timeOut; try { //return _serialPort.BaseStream.ReadByte(); return _serialPort.ReadByte(); // _serialPort.Read(array, 0, 1); // return array[0]; } catch (TimeoutException) { return -1; } } public int ReadBytes(byte[] array, int length, int timeOut = 200) { if (timeOut != _readTimeOut) _serialPort.ReadTimeout = _readTimeOut = timeOut; try { //return _serialPort.BaseStream.ReadByte(); int bytesRead = 0; while ( bytesRead < length ) bytesRead += _serialPort.Read(array, bytesRead, length - bytesRead); // _serialPort.Read(array, 0, 1); // return array[0]; return bytesRead; } catch (TimeoutException) { return -1; } } /// <summary> /// sends string followed by CR - LF /// </summary> /// <param name="line"></param> public void WriteLine(String line) { WriteBytes(StringToBytes(line + "\r\n")); } public static byte[] StringToBytes(string input) { return Encoding.ASCII.GetBytes(input); } public void Close() { try { _serialPort.DtrEnable = false; _serialPort.RtsEnable = false; _serialPort.Close(); } catch(IOException) { } } public bool Dtr { get { return _serialPort.DtrEnable; } set { _serialPort.DtrEnable = value; } } public bool Rts { get { return _serialPort.RtsEnable; } set { _serialPort.RtsEnable = value; } } } }
-
Admin over 10 yearsThanks this was execly what i was looking for, Thank you very much. I will accept this as anwser asap
-
Admin over 10 yearsAn quick question about this, i have multiple textboxes so how can i put them in var content
-
dbasnett over 10 yearsThe default Newline used by WriteLine is LF(\n) only.
-
user2586804 over 10 years@dbasnett Their
WriteLine
, i.e.ComPort.WriteLine
from question:WriteBytes(StringToBytes(line + "\r\n"));
-
user2586804 over 10 yearsKoornstra so many ways. I'd write a new method on comport called
WriteLines
that takes anIEnumerable<string>
. Formats them into a single line with '\r\n' after each line and sends that as one.