Serialization byte array vs XML file
Solution 1
This should be fine, but you're doing work that is already done for you. Look at the System.Runtime.Serialization.Formatters.Binary.BinaryFormatter
class.
Rather than needing to implement your own Read/WriteOjbectData() methods for each specific type you can just use this class that can already handle most any object. It basically takes an exact copy of the memory representation of almost any .Net object and writes it to or reads it from a stream:
BinaryFormatter bf = new BinaryFormatter();
bf.Serialize(outputStream, objectToSerialize);
objectToDeserialize = bf.Deserialize(inputStream) as DeserializedType;
Make sure you read through the linked documents: there can be issues with unicode strings, and an exact memory representation isn't always appropriate (things like open Sockets, for example).
Solution 2
If you are after simple, lightweight and efficient binary serialization, consider protobuf-net; based on google's protocol buffers format, but implemented from scratch for typical .NET usage. In particular, it can be used either standalone (via protobuf-net's Serializer
), or via BinaryFormatter
by implementing ISerializable
(and delegating to Serializer
).
Apart from being efficient, this format is designed to be extensible and portable (i.e. compatible with java/php/C++ "protocol buffers" implementations), unlike BinaryFormatter that is both implementation-specific and version-intolerant. And it means you don't have to mess around writing any serialization code...
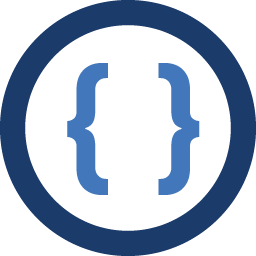
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I am heavily using byte array to transfer objects, primitive data, over the network and back. I adapt java's approach, by having a type implement ISerializable, which contains two methods, as part of the interface, ReadObjectData and WriteObjectData. Any class using this interface, would write date into the byte array. Something Like that
class SerializationType:ISerializable { void ReadObjectData (/*Type that manages the write/reads into the byte array*/){} void WriteObjectData(/*Type that manages the write/reads into the byte array*/){} }
After write is complete for all object, I send an array of the network.
This is actually two-fold question. Is it a right way to send data over the network for the most efficiency (in terms of speed, size)?
Would you use this approach to write objects into the file, as opposed to use typically xml serialization?
Edit #1
Joel Coehoorn mentioned BinaryFormatter. I have never used this class. Would you elaborate, provide good example, references, recommendations, current practices -- in addition to what I currently see on msdn?
-
Admin over 15 yearsGood Idea. I suppose for storing file, a xml file is a good idea. As for the wire transfer, specific to my case, i cannot use xml, as the other end receiving data is C++ server that only reads and writes data one way, and one way alone -- to/from byte array. Thanks
-
Admin over 15 yearsWhen was ISerializationFactory introduced? ISerializable is an internal interface, so I can easily modify all serialization types.... I will take a note of it. Thanks
-
Admin over 15 yearsEffective Java is a great book -- I read both edition cover to cover!
-
Admin over 15 yearsThat may not work well if a specific layout in the binary array is required.
-
Joel Coehoorn over 15 yearsThat's right: it does assume .Net at both ends, or that any non-.Net participant will parse the .Net format.
-
Admin over 15 yearslooks good [for personal use]. I'm not sure I want to include it into commercial code, until at least I fully understand license guidelines. It only facilitates file serialization. I need something for network too. thanks
-
Marc Gravell over 15 yearsBinaryFormatter is implementation-specific, version-intolerant, and not especially quick for large graphs - but it is, of course, perfectly functional for .net-to-.net. Personally, I'd go for protobuf-net, of course ;-p
-
01es over 15 yearsWe're using it specifically for network communication (as a way to communicate between a REST service and a client). A two minute tutorial [xstream.codehaus.org/tutorial.html] shows that one can simply write String xml = xstream.toXML(joe); and then do with xml what ever is needed.