Sessions in ZF2
Solution 1
You don't need to do any configuration to use sessions in Zend Framework 2. Sure, you can change settings, but if you just want to get up and running with sessions, then don't worry about it for now.
My apologies, but I am going to disregard your last sentence; about a month ago, I wrote an article about this subject with the purpose of showing how to quickly get started with using sessions in ZF2. It doesn't rank well in search engines, so chances are you haven't read it.
Here is a code snippet showing how it can be done. If you are interested in how it works behind the scenes, then please refer to the link above.
namespace MyApplication\Controller;
use Zend\Mvc\Controller\AbstractActionController;
use Zend\Session\Container; // We need this when using sessions
class UserController extends AbstractActionController {
public function loginAction() {
// Store username in session
$userSession = new Container('user');
$userSession->username = 'Andy0708';
return $this->redirect()->toRoute('welcome');
}
public function welcomeAction() {
// Retrieve username from session
$userSession = new Container('user');
$username = $userSession->username; // $username now contains 'Andy0708'
}
}
Solution 2
about session
Where do you want to store session (in mysql-db, in mongo-db,or in ram,..)? How to classify session into "namespace" like
$_SESSION["namespace"]["user"],
$_SESSION["namespace_1"]["user"]?
SessionManager
in Zend, Zend\Session\SessionManger help you to do many as what listed above
simple config for SessionManger
$sessionManager = new SessionManager();
$sessionStorage = new SessionArrayStorage();
$sessionManager->setStorage($sessionStorage);
//you can add more config, read document from Zend
$sessionContainer = new Container("ABC", $sessionManager);
$sessionContainer->offsetSet("user", "lehoanganh25991");
when you call new Container without any SessionManager config
$sessionContainer = new Container("abc");
behind the scence, Zend create a default SessionManager, then pass it into Container
SessionArrayStorage, $_SESSION
SessionArrayStorage in Zend can work with $_SESSION, we can access to user
above through
$_SESSION["ABC"]["user"]
if you set other storages like mysql-db, mongo-db, in ram,.. access through $_SESSION may not work
access session
in Module A, set session
How can we access it in Moudle B? where $sessionContainer variable @@?
it quite weird, but when you want to access to the this container, create a new one with SAME CONFIG
$sessionManager = new SessionManager();
$sessionStorage = new SessionArrayStorage();
$sessionManager->setStorage($sessionStorage);
$sessionContainer = new Container("ABC", $sessionManager);
//access
var_dump("get user from \$sessionContainer", $sessionContainer->offsetGet("user"));
review demo on github: https://github.com/hoanganh25991/zend-auth-acl/tree/d0a501e73ac763d6ef503bbde325723ea1868351
(through commits, the project changed, access it at this tree)
in FronEnd\Controller\IndexController
in AuthAcl\Module.php
in AuthAcl\Service\SimpleAuth
i acces same session at different places
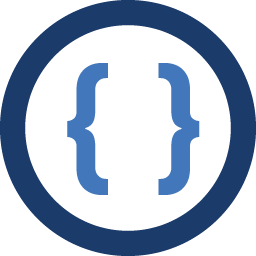
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
Could you tell me how to properly use sessions in ZF2? So far I have this code:
"session" => [ "remember_me_seconds" => 2419200, "use_cookies" => true, "cookie_httponly" => true ]
That's the session config I copied from some post here on stackoverflow. Now should I put this code into module.config.php in each module that uses sessions or in the Application module?
public function onBootstrap(EventInterface $Event) { $Config = $Event->getApplication()->getServiceManager()->get('Configuration'); $SessionConfig = new SessionConfig(); $SessionConfig->setOptions($Config['session']); $SessionManager = new SessionManager($SessionConfig); $SessionManager->start(); Container::setDefaultManager($SessionManager); }
Same problem with the onBootstrap() method of the Module class. Should this code go into each module's Module class or just once into the Application's Module class?
In both cases I have tried both approaches and I even tried putting this code into both modules at once, but the only thing I was able to accomplish was to set session variables in controller's constructor and then read them in actions/methods. I wasn't able to set a session variable in one action/method and then read it in another. If I remove the lines in which I set the variables in controller's constructor, I can no longer see these variables in the session. The session just behaves like it was created and deleted each time a page is requested.
Am I missing something? Please don't link me to any resources on the internet, I have read them all and they're not really helpful.