set checkbox in gridview based on datatable value
Solution 1
You can do it like this:
First in sql server:
SELECT
CAST(CASE PROCESSED WHEN 'Y' THEN 1 ELSE 0 END AS BIT) AS PROCESSED
NAME
DATE
FROM ExampleTable
in c# code:
SqlCommand cmd = new SqlCommand(sql query here);
SqlDataAdapter da = new SqlDataAdapter();
DataTable dt = new DataTable();
da.SelectCommand = cmd;
// Save results of select statement into a datatable
da.Fill(dt);
gridview_all_applicants.DataSource = dt;
gridview_all_applicants.DataBind();
and finally in aspx:
<asp:TemplateField HeaderText="Complete">
<ItemTemplate>
<asp:CheckBox ID="process_flag" runat="server" Checked='<%# bool.Parse(Eval("PROCESSED").ToString()) %>' Enable='<%# !bool.Parse(Eval("PROCESSED").ToString()) %>'/>
</ItemTemplate>
Solution 2
How about a database agnostic solution, just on the off chance you are using a non sqlserver database ;)
<asp:CheckBox ID="process_flag_check" runat="server"
Checked='<%# Eval("process_flag").ToString() == "Y" ? "True": "False" %>'
Enabled="false"/>
</asp:Content>
Solution 3
<asp:CheckBox ID="process_flag_check" runat="server"
Checked='<%# bool.Parse(Eval("process_flag").ToString() == "Y" ? "True": "False") %>'
Enabled="false"/>
</asp:CheckBox>
This one Perfectly Works.I have modified using Kevin and marco Code. Thank u Kevinv and Marco
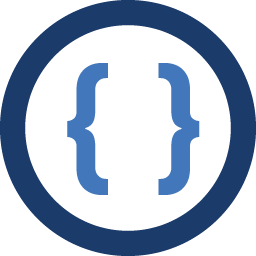
Admin
Updated on July 17, 2022Comments
-
Admin almost 2 years
I have a gridview control with a checkbox field and several bound fields. The checkbox field does not directly map to a field in the database. Rather, i want to read a value from a field in the database and "check" some of the checkboxes.
For example, given the following data from the database -> datatable
PROCESSED NAME DATE Y Mickey Mouse 11/15/2011 N Donald Duck 4/01/2012 Y James Bond 5/02/2011
I would like the gridview to display a checkbox and set the value of boxes to UNCHECKED where PROCESSED = N and for PROCESSED = Y either have an uneditable checkbox or no checkbox at all.
PROCESSED NAME DATE [/] Mickey Mouse 11/15/2011 [ ] Donald Duck 4/01/2012 [/] James Bond 5/02/2011
To populate the gridview, a SQL stmt is run against a database, and the result of the SQL query is stored in a datatable. Before binding the datatable to the gridview, i would like to check the "processed" field and set the checkbox based on that value.
Here is the gridview control (shortened for clarity):
<asp:GridView ID="gridview_all_applicants" runat="server" AllowPaging="True"> <Columns> <asp:TemplateField HeaderText="Complete"> <ItemTemplate> <asp:CheckBox ID="process_flag" runat="server" /> </ItemTemplate> </asp:TemplateField> <asp:BoundField DataField="lastname" HeaderText="Last Name" ReadOnly="True" SortExpression="lastname" />
Here is what i have so far in the code behind
SqlCommand cmd = new SqlCommand(sql query here); SqlDataAdapter da = new SqlDataAdapter(); DataTable dt = new DataTable(); da.SelectCommand = cmd; // Save results of select statement into a datatable da.Fill(dt); foreach (DataRow r in dt.Rows) { // do some processing of data returned from query // read the char value from the returned data and set checkbox procflag = r["process_flag"].ToString().ToLower(); CheckBox chkbox = new CheckBox(); if (procflag == null || procflag == "n") { // SET CHECKBOX TO "NOT CHECKED" } else { // SET CHECKBOX TO "CHECKED" AND MAKE IT UNCLICKABLE // ----OR---- DO NOT DISPLAY CHECKBOX AT ALL. } } // end for each gridview_all_applicants.DataSource = dt; gridview_all_applicants.DataBind();
any help is greatly appreciated.
-
Admin almost 13 yearsMarco - you my friend are an absolute GENIUS! What an elegant solution....works perfect.....THANK YOU!!!