Set Label Text with JQuery
121,704
Solution 1
The checkbox is in a td
, so need to get the parent first:
$("input:checkbox").on("change", function() {
$(this).parent().next().find("label").text("TESTTTT");
});
Alternatively, find a label which has a for
with the same id
(perhaps more performant than reverse traversal) :
$("input:checkbox").on("change", function() {
$("label[for='" + $(this).attr('id') + "']").text("TESTTTT");
});
Or, to be more succinct just this.id
:
$("input:checkbox").on("change", function() {
$("label[for='" + this.id + "']").text("TESTTTT");
});
Solution 2
I would just query for the for
attribute instead of repetitively recursing the DOM tree.
$("input:checkbox").on("change", function() {
$("label[for='"+this.id+"']").text("TESTTTT");
});
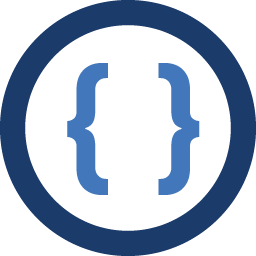
Author by
Admin
Updated on January 07, 2020Comments
-
Admin over 4 years
This should be quite straight forward, however the following code does not do anything as far as changing the next label's text. I have tried using .text, .html, and so on to no avail. Is there anything wrong with this code?
<script type="text/javascript"> $(document).ready(function() { $("input:checkbox").on("change", checkboxChange); function checkboxChange() { $("#"+this.id).next("label").text("TESTTTT"); } }); </script> <td width="15%" align="center"><input type="checkbox" name="task1" id="task1"></td> <td width="25%" align="center"><label for="task1"></label></td>
-
A. Wolff over 10 years@BrettPowell you should use FOR attribute of label instead
-
CodingIntrigue over 10 years
this.id
is fine, no need to wrap it as a jQuery object -
Abhitalks over 10 years@A.Wolff: yes, i inittially wanted to show the problem and was editing the answer to show with for.
-
A. Wolff over 10 yearsSo now you have a complete answer +1
-
sun almost 10 yearsThis work good. Do you refer any books to know about this kind of selector and functions
-
Abhitalks almost 10 years@sun: you may want to start with this: learningjquery.com although not a book, but the entire site is available as a 5mb download if you wish.