Set object property using reflection
Solution 1
Yes, you can use Type.InvokeMember()
:
using System.Reflection;
MyObject obj = new MyObject();
obj.GetType().InvokeMember("Name",
BindingFlags.Instance | BindingFlags.Public | BindingFlags.SetProperty,
Type.DefaultBinder, obj, "Value");
This will throw an exception if obj
doesn't have a property called Name
, or it can't be set.
Another approach is to get the metadata for the property, and then set it. This will allow you to check for the existence of the property, and verify that it can be set:
using System.Reflection;
MyObject obj = new MyObject();
PropertyInfo prop = obj.GetType().GetProperty("Name", BindingFlags.Public | BindingFlags.Instance);
if(null != prop && prop.CanWrite)
{
prop.SetValue(obj, "Value", null);
}
Solution 2
You can also do:
Type type = target.GetType();
PropertyInfo prop = type.GetProperty("propertyName");
prop.SetValue (target, propertyValue, null);
where target is the object that will have its property set.
Solution 3
Reflection, basically, i.e.
myObject.GetType().GetProperty(property).SetValue(myObject, "Bob", null);
or there are libraries to help both in terms of convenience and performance; for example with FastMember:
var wrapped = ObjectAccessor.Create(obj);
wrapped[property] = "Bob";
(which also has the advantage of not needing to know in advance whether it is a field vs a property)
Solution 4
Or you could wrap Marc's one liner inside your own extension class:
public static class PropertyExtension{
public static void SetPropertyValue(this object obj, string propName, object value)
{
obj.GetType().GetProperty(propName).SetValue(obj, value, null);
}
}
and call it like this:
myObject.SetPropertyValue("myProperty", "myValue");
For good measure, let's add a method to get a property value:
public static object GetPropertyValue(this object obj, string propName)
{
return obj.GetType().GetProperty(propName).GetValue (obj, null);
}
Solution 5
Use somethings like this :
public static class PropertyExtension{
public static void SetPropertyValue(this object p_object, string p_propertyName, object value)
{
PropertyInfo property = p_object.GetType().GetProperty(p_propertyName);
property.SetValue(p_object, Convert.ChangeType(value, property.PropertyType), null);
}
}
or
public static class PropertyExtension{
public static void SetPropertyValue(this object p_object, string p_propertyName, object value)
{
PropertyInfo property = p_object.GetType().GetProperty(p_propertyName);
Type t = Nullable.GetUnderlyingType(property.PropertyType) ?? property.PropertyType;
object safeValue = (value == null) ? null : Convert.ChangeType(value, t);
property.SetValue(p_object, safeValue, null);
}
}
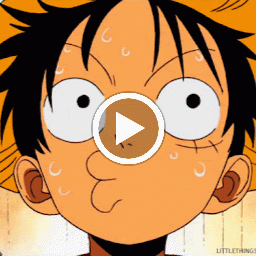
Renan Rodrigues
Updated on July 08, 2022Comments
-
Renan Rodrigues almost 2 years
Is there a way in C# where I can use reflection to set an object property?
Ex:
MyObject obj = new MyObject(); obj.Name = "Value";
I want to set
obj.Name
with reflection. Something like:Reflection.SetProperty(obj, "Name") = "Value";
Is there a way of doing this?
-
Shivang Gangadia about 15 yearsI have done this very same thing today. The above works great, obviously a null check should be done on prop before attempting to use it.
-
halfpastfour.am over 12 yearsWow, got a little confused from the merge, but i found your answer again! Thank you, you deserve an 'accept', but since my thread is merged :( Thanks again!
-
LostNomad311 almost 12 yearsIf you aren't dealing with all strings you might wanna convert the data first:
var val = Convert.ChangeType(propValue, propInfo.PropertyType);
source: devx.com/vb2themax/Tip/19599 -
Sudhanshu Mishra over 10 years@MarcGravell, I was looking at FastMember and it is pretty interesting. Is there a getting started/tutorial somewhere for us mere mortals to use this great lib of yours?
-
ThreeFx over 9 yearsHi and welcome to Stack Overflow. Please format your code properly and not just dump it in your post, it will help others understand your answer.
-
x19 over 9 yearsHow can I get the type of property by FastMember?
-
Marc Gravell over 9 years@Jahan accessor => GetMembers => Member => Type
-
j.i.h. over 9 years@AntonyScott I would think you'd want to know if you're invoking the wrong property, so "fail silently" seems like a bad course.
-
Shivang Gangadia over 9 years@j.i.h. I can see your point, but it depends on the situation really.
-
Marc over 8 yearsThe part where you get the property type and then cast it was really useful for me. It works like a charm. Thank you
-
Bastiaan almost 8 yearsgreat answer! The good thing about a oneliner is that it is much faster to understand, since there are no user named variables in between which for yourself might not make any sense..
-
tecfield almost 7 yearsalternatively, you can use
obj.GetType().GetProperty("Name")?.GetSetMethod()?.Invoke(...)
-
T.Todua about 6 yearsits impossible to set value for
CanWrite=False
types, right?