Set span for items in GridLayoutManager using SpanSizeLookup
60,195
Solution 1
The problem was that header should have span size of 2, and regular item should have span size of 1. So correct implementations is:
mLayoutManager.setSpanSizeLookup(new GridLayoutManager.SpanSizeLookup() {
@Override
public int getSpanSize(int position) {
switch(mAdapter.getItemViewType(position)){
case MyAdapter.TYPE_HEADER:
return 2;
case MyAdapter.TYPE_ITEM:
return 1;
default:
return -1;
}
}
});
Solution 2
Header should have a span equal to the span count of the entire list.
mLayoutManager.setSpanSizeLookup(new GridLayoutManager.SpanSizeLookup() {
@Override
public int getSpanSize(int position) {
switch(mAdapter.getItemViewType(position)){
case MyAdapter.TYPE_HEADER:
return mLayoutManager.getSpanCount();
case MyAdapter.TYPE_ITEM:
return 1;
default:
return -1;
}
}
});
Solution 3
Answer to my own question: Override the getSpanSizeLookup() from the Activity after setting the adapter.
Related videos on Youtube
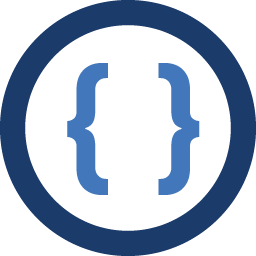
Author by
Admin
Updated on November 19, 2020Comments
-
Admin over 3 years
I want to implement grid-like layout with section headers. Think of https://github.com/TonicArtos/StickyGridHeaders
What I do now:
mRecyclerView = (RecyclerView) view.findViewById(R.id.grid); mLayoutManager = new GridLayoutManager(getActivity(), 2); mLayoutManager.setSpanSizeLookup(new GridLayoutManager.SpanSizeLookup() { @Override public int getSpanSize(int position) { switch(mAdapter.getItemViewType(position)){ case MyAdapter.TYPE_HEADER: return 1; case MyAdapter.TYPE_ITEM: return 2; default: return -1; } } }); mRecyclerView.setLayoutManager(mLayoutManager);
Now both regular items and headers have span size of 1. How do I solve this?
-
yigit over 9 yearsthis implementation looks correct to me. Did you debug if your
mAdapter.getItemViewType(position)
is returning the correct value ? -
BladeCoder over 7 years"1" seems like a safer default value than "-1".
-
Vijay Ram about 4 yearsI am a newbie. For me, this link helped me 3 RecyclerView Infinite Scroll Examples
-
-
Karthik Rk over 8 yearsget span size method determines the amount span width your cell is going to take not the number of col row should have !!
-
Ronny Shibley about 7 yearswhen spanning the first item, it's messing up the height of the next ones. It works on any other item. Any idea ?
-
Umair over 6 years@RonnyShibley any solution for the issue you stated above ... I am also facing the same issue, first item after the header doesn't shows , others are all shown up as required
-
Mahdi over 5 yearsThis is not woking.
-
Bruno Bieri over 2 yearsHow comes no one links to the actual documentation: developer.android.com/reference/kotlin/androidx/recyclerview/… Hence, this answer seems more accurate to me then the currently accepted one. Maybe create a const variable with the span count and use it for the
GridLayoutManager
constructor and in thegetSpanSize
method to avoid thegetSpanCount
call for every header.