Show menu after 2 seconds
Solution 1
Use a timeout rather than .delay()
, the latter only affects queued functions (such as effects):
$("#dropdown").hover(function() {
setTimeout(function() {
$(".drop-inner").show();
}, 2000);
});
Solution 2
You can use javascripts setTimeout
function to delay execution:
<script>
$(document).ready(function() {
$("#dropdown").hover(function() {
setTimeout(function() {
$(".drop-inner").show()
}, 2000);
});
});
</script>
Solution 3
It doesn't seem like .show()
will work with .delay()
:
Added to jQuery in version 1.4, the .delay() method allows us to delay the execution of functions that follow it in the queue. It can be used with the standard effects queue or with a custom queue. Only subsequent events in a queue are delayed; for example this will not delay the no-arguments forms of .show() or .hide() which do not use the effects queue.
Solution 4
I agree with the setTimeout
solution, but ... what if the user moves away mouse cursor from the #dropdown
before those 2 seconds elapses?
<script>
$(document).ready(function() {
$("#dropdown").hover(function(){
$.data(this, "timer", setTimeout($.proxy(function() {
$(".drop-inner").show();
}, this), 2000));
},
function() {
clearTimeout($.data(this, "timer"));
$(".drop-inner").hide();
});
});
</script>
Check the example on http://jsfiddle.net/vundicind/Lqq3h1xa/1/.
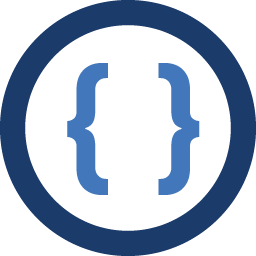
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
Im trying having problems on showing a
<div>
after 2 seconds.. My problem is that when i hover the button, the menu is displayed with no delay, and i want a delay of 2 secs...Try to hover: Mere på cabiweb, then the menu will display -> You can see it here
The code :
<script>$(document).ready(function() { $("#dropdown").hover(function(){ $(".drop-inner").delay(2000).show(); }); });</script>