Simple Binding of Data from code behind to XAML
Databinding does not work with fields. Use Properties instead:
public int TmpVal {get; set;}
public string TmpStr {get; set;}
Also if you want the textbox to automatically pick up changes from your data you would ideally need to implement INotifyPropertyChanged or make it a dependency property or have a XXXChanged event for each XXX property (this doesn't work anymore).
<Window x:Class="WpfApplication5.Window1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="Window1" Height="300" Width="300" x:Name="ThisWindow">
<StackPanel>
<TextBox Name="txtTest1" Text="{Binding TmpStr, ElementName=ThisWindow}" />
<Button Name="butTest1" Click="viewButton_Click">Test123</Button>
</StackPanel>
</Window>
And the code behind:
public partial class Window1 : Window, INotifyPropertyChanged
{
public Window1()
{
this.TmpStr = "Windows Created";
this.InitializeComponent();
this.DataContext = this;
}
public event PropertyChangedEventHandler PropertyChanged;
public string TmpStr { get; set; }
public int TmpVal { get; set; }
private void viewButton_Click(object sender, RoutedEventArgs args)
{
this.TmpStr = "Button clicked";
if (this.PropertyChanged != null)
{
this.PropertyChanged(this, new PropertyChangedEventArgs("TmpStr"));
}
}
}
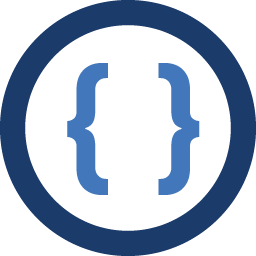
Admin
Updated on June 04, 2022Comments
-
Admin almost 2 years
I am new to WPF concepts. I want to just display a string in a textbox. I tried the following C# code and XAML to bind a string to a TextBox.Text property. C# code:public partial class Window1 : Window { public int TmpVal; public string TmpStr; public Window1() { TmpVal = 50; TmpStr = "Windows Created"; InitializeComponent(); this.DataContext = this; } private void viewButton_Click(object sender, RoutedEventArgs args) { TmpStr = "Button clicked"; } }
}
XAML:
<Window x:Class="TestWPF.Window1" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Title="Window1" Height="600" Width="800" x:Name="ThisWindow"> <Grid> <TextBox Name="txtTest1" Margin="200,0,200,200" HorizontalAlignment="Left" Height="50" Width="200" Text="{Binding TmpStr, ElementName=ThisWindow}" /> <Button Name="butTest1" Click="viewButton_Click">Test123</Button> </Grid> </Window>
On execution I always get blank text in my textbox (even when I invoke the click event).
I browsed through the stackoverflow site but couldn't solve the problem (though many questions were close to this one)
Can someone suggest me if anything is overlooked or missed out? -
SMART_n over 14 yearsWhy XXXChanged events doesn't work anymore? Where can I read about this?
-
Erusso87 over 14 yearsI think is was only there in some CTPs or early betas of v1.
-
ChrisF over 14 yearsYou should implement the
PropertyChanged
code in the Property Setter. Thus if your property gets changed by code the UI will update. -
Erusso87 over 14 yearsI took this for granted. I just wanted to display as little code as possible.
-
Jonathan Brown almost 9 yearsTHANK YOU! "Databinding does not work with fields. Use Properties instead"