Simple login function in Python
Solution 1
You call your login()
function two times. Only the second one utilizes the return value, so you can remove the first call
def main():
login() # <--- Remove this one
log = login()
Something you may wish to consider using is getpass
. Using this instead of raw_input
on your password field will prevent the password from being displayed on screen.
import getpass
...
def login():
...
passw = getpass.getpass("Password: ")
The rest of the code then behaves the same, but on the console, the output looks like this:
Username: max2
Password:
Login successful!
Notice that the "Password:" line is empty, despite typing in a valid password
Solution 2
The problem here is that you are calling login()
twice whitin main
. To fix your bug, just remove the first call since the return is not even used. Also, I'm not sure whether it's intended but:
if (user in us) and (passw in pw):
will match ('user', 'pass') even if (us, pw) is ('username', 'password'). Use ==
operator instead.
One least thing, you should consider changing:
us, pw = line.strip().split("|")
To:
us, pw = line.strip().split("|", 1)
Which splits the line only once, otherwise the passwords won't be able to contain |
Solution 3
The issue was that there were two instances of login() being called. The following Code Will Repeat The Login until it is correct:
def login():
user = raw_input("Username: ")
passw = raw_input("Password: ")
f = open("users.txt", "r")
for line in f.readlines():
us, pw = line.strip().split("|")
if (user in us) and (passw in pw):
print "Login successful!"
return True
print "Wrong username/password"
return False
def menu():
#here's a menu that the user can access if he logged in.
def main():
global True
while True:
True = True
log = login()
if log == True:
menu()
True = False
main()
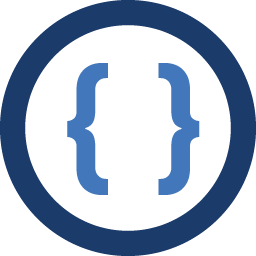
Admin
Updated on June 09, 2022Comments
-
Admin almost 2 years
def login(): user = raw_input("Username: ") passw = raw_input("Password: ") f = open("users.txt", "r") for line in f.readlines(): us, pw = line.strip().split("|") if (user in us) and (passw in pw): print "Login successful!" return True print "Wrong username/password" return False def menu(): #here's a menu that the user can access if he logged in. def main(): login() log = login() if log == True: menu()
And the users.txt is:
john22|1234 max2|2211 jack6|1551
The problem is, when I run the program and enter the correct Username and Password, it prints out "Login successful!" but it doesn't continue to menu(). Instead, it shows "Username: " and "Password: " again. However, when I enter the correct username and password again (same or different pair from users.txt), it enters the menu() and everything works fine. So my guess is that you have to, for some reason, enter the correct info twice, and I don't want that. I searched everywhere but I can't figure out why is this happening...