Simple Rest Webservice returning http status 404
Sometimes troubleshooting java webservices is frustrating so it is helpful to follow some simple steps to find the error.
Is your firewall on? Check that it is off or not blocking the port your webserver running on. Most of the time it is port 8080. It is in the url localhost:
8080
/mywebservice/urlCheck the root application page supplied by your server to make sure the server is running at all. On Tomcat that usually localhost:8080/manager/html
Check the logs of your application container for a stacktrace on startup to make sure your deployment is not in error.
Try to deploy a minimum possible webservice such as "Hello World" jax-rs to make sure you have the correct libraries and other configurations available.
-
If you pass all of those and still no webservice your jax-rs annotations are probably incorrect in some manner. You can enable Request Matching in your web.xml by adding to the configuration.
<web-app> <servlet> <servlet-name>Jersey REST Service for value codes</servlet-name> <servlet-class>com.sun.jersey.spi.container.servlet.ServletContainer</servlet-class> ... <init-param> <param-name>com.sun.jersey.config.feature.Trace</param-name> <param-value>true</param-value> </init-param> </servlet> ... </web-app>
You'll see url traces in your log
{X-Jersey-Trace-008=[mapped exception to response: javax.ws.rs.WebApplicationException@56f9659d -> 415 (Unsupported Media Type)],
X-Jersey-Trace-002=[accept right hand path java.util.regex.Matcher[pattern=/myResource/([-0-9a-zA-Z_]+)(/.*)? region=0,17 lastmatch=/myResource/23/mySubresources]: "/myResource/23/mySubresources" -> "/myResource/23" : "/mySubresources"],
hint: probably not a good idea to leave this on in production
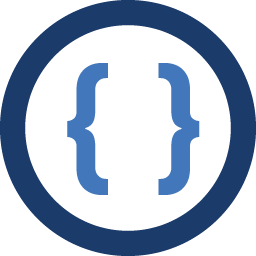
Admin
Updated on June 13, 2022Comments
-
Admin almost 2 years
I have been trying to get this tutorial to work: Link I am using Apache Tomcat 7.0 and the Jersey 2.0 libraries. This is my service:
package org.arpit.javapostsforlearning.webservice; import javax.ws.rs.GET; import javax.ws.rs.Path; import javax.ws.rs.PathParam; import javax.ws.rs.Produces; import javax.ws.rs.core.MediaType; @Path("ConversionService") public class FeetToInchAndInchToFeetConversionService { @GET @Path("/InchToFeet/{i}") @Produces(MediaType.TEXT_XML) public String convertInchToFeet(@PathParam("i") int i) { int inch=i; double feet = 0; feet =(double) inch/12; return "<InchToFeetService>" + "<Inch>" + inch + "</Inch>" + "<Feet>" + feet + "</Feet>" + "</InchToFeetService>"; } @Path("/FeetToInch/{f}") @GET @Produces(MediaType.TEXT_XML) public String convertFeetToInch(@PathParam("f") int f) { int inch=0; int feet = f; inch = 12*feet; return "<FeetToInchService>" + "<Feet>" + feet + "</Feet>" + "<Inch>" + inch + "</Inch>" + "</FeetToInchService>"; } }
and this is my web.xml:
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <display-name>RESTfulWebServiceExample</display-name> <servlet> <servlet-name>Jersey REST Service</servlet-name> <servlet-class>org.glassfish.jersey.servlet.ServletContainer</servlet-class> <init-param> <param-name>jersey.config.server.provider.packages</param-name> <param-value>org.arpit.javapostsforlearning.webservice</param-value> </init-param> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>Jersey REST Service</servlet-name> <url-pattern>/rest/*</url-pattern> </servlet-mapping> </web-app>
I tried to Run it on server to deploy and I also tried to let eclipse export it as a war file and then deploy it with the tomcat application manager. Both ways I get the HTTP Status 404, The requested resource is not available. Prior to this there is error message in any logs. I have also tried to put a simple index.html file in the Webcontent folder, but I could also not access that in the browser. I know that there are a lot of similar posts on the forum, but after having read them and hours of trying i still cannot figure out how to solve my problem.