Simulate Enter In JavaScript
Solution 1
For anyone that has a problem dispatching the event, try adding the key-value pair bubbles: true
:
const keyboardEvent = new KeyboardEvent('keydown', {
code: 'Enter',
key: 'Enter',
charCode: 13,
keyCode: 13,
view: window,
bubbles: true
});
Solution 2
var textArea = document.getElementById('text');
function enter() {
var keyboardEvent = new KeyboardEvent('keydown', {
code: 'Enter',
key: 'Enter',
charKode: 13,
keyCode: 13,
view: window
});
textArea.dispatchEvent(keyboardEvent);
}
textArea.addEventListener('keydown', function (e) {
console.log(e);
});
enter()
<!doctype html>
<html class="no-js" lang="">
<head>
<meta charset="utf-8">
<meta http-equiv="x-ua-compatible" content="ie=edge">
<title>test</title>
<meta name="description" content="">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
</head>
<body>
<textarea id="text"></textarea>
</body>
</html>
At this moment initKeyboardEvent
method is marked as deprecated. It is recommended to instantiate KeyboardEvent
directly and pass necessary data to constructor - link. See the browser compatibility section. At least it works in chrome and firefox.
Solution 3
I think it's a browser bug since keyboardEvent.which
is unwritable. In order to fix it, you have to delete keyboardEvent.which
property before assigning the keycode.
function enter1() {
var keyboardEvent = document.createEvent('KeyboardEvent');
delete keyboardEvent.which;
var initMethod = typeof keyboardEvent.initKeyboardEvent !== 'undefined' ? 'initKeyboardEvent' : 'initKeyEvent';
keyboardEvent[initMethod](
'keydown', // event type : keydown, keyup, keypress
true, // bubbles
true, // cancelable
window, // viewArg: should be window
false, // ctrlKeyArg
false, // altKeyArg
false, // shiftKeyArg
false, // metaKeyArg
13, // keyCodeArg : unsigned long the virtual key code, else 0
13 // charCodeArgs : unsigned long the Unicode character associated with the depressed key, else 0
);
document.getElementById('text').dispatchEvent(keyboardEvent);
}
An alternative solution is KeyboardEvent Constructor. Just be careful with the compatibility issue.
function enter1() {
var keyboardEvent = new KeyboardEvent('keydown');
delete keyboardEvent.which;
keyboardEvent.which = 13;
document.getElementById('text').dispatchEvent(keyboardEvent);
}
Related videos on Youtube
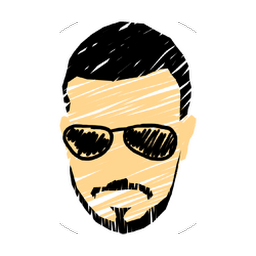
Luca Laiton
Updated on July 18, 2022Comments
-
Luca Laiton almost 2 years
I try to simulate
Enter
inJavaScript
in a specificTextArea
. This is my code:function enter1() { var keyboardEvent = document.createEvent('KeyboardEvent'); var initMethod = typeof keyboardEvent.initKeyboardEvent !== 'undefined' ? 'initKeyboardEvent' : 'initKeyEvent'; keyboardEvent[initMethod]('keydown', // event type : keydown, keyup, keypress true, // bubbles true, // cancelable window, // viewArg: should be window false, // ctrlKeyArg false, // altKeyArg false, // shiftKeyArg false, // metaKeyArg 13, // keyCodeArg : unsigned long the virtual key code, else 0 13 // charCodeArgs : unsigned long the Unicode character associated with the depressed key, else 0 ); document.getElementById('text').dispatchEvent(keyboardEvent); }
TextArea
:<textarea id="text"> </textarea>
When I call enter1(), it doesn't do anything in the
TextArea
. Why is this? -
Luca Laiton about 9 yearsi edited the code, but nothing changed. thanks for your answer.
-
Lewis about 9 years@LucaLaiton You can try manually assigning
keyboardEvent.which = 13;
instead of passing keycode intoinitMethod
. -
Luca Laiton about 9 years<pre> <code> function premi_enter() { var keyboardEvent = document.createEvent('KeyboardEvent'); delete keyboardEvent.which; keyboardEvent.which = 13; document.getElementById('text').dispatchEvent(keyboardEvent); } </pre> </code>
-
Lewis about 9 years@LucaLaiton Yes. BTW, I've updated my answer with a more elegant solution. I thought you may be interested in it.
-
Luca Laiton about 9 yearsthanks for support, but the last one function doesn't work for compatibility, maybe. I need only simulate the pressing of enter key, for me is very hard :)
-
Reem over 6 yearsI have the same problem and I still can't get that working
-
SFin almost 6 yearsThis approach looks obsolete now. developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/keyCode
-
C.M. almost 4 years@SFin I removed the charKode and keyCode properties and this solution worked for me.
-
Shehroz Ahmed almost 3 yearsWorked for me, Thanks!