Skip for loop when if statement is met inside for loop
10,002
Solution 1
You could use the break keyword.
for (int i = 0; i < 100; i++){
if (i == 2){
break;
}
}
Also, this is considered by OOD people a gotoish code, prefer inserting the break condition in the for condition :
boolean shouldBreak = false;
for (int i = 0; i < 100 && !shouldBreak; i++){
if (i == 2){
shouldBreak = true;
}
}
Solution 2
Use the break;
keyword to do that.
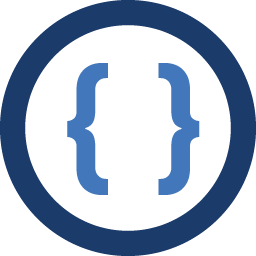
Author by
Admin
Updated on June 04, 2022Comments
-
Admin about 2 years
I have an
if
statement inside afor
loop. When the the condition inif
statement is true, I want to break out of thefor
loop.here is what exactly I have
if (data < voltage && data2 < voltage) { digitalWrite(pump, HIGH); digitalWrite(valve, HIGH); digitalWrite(valve2, HIGH); for (int i = 0; i < 10; i++) { lcd.setCursor(0, 2); lcd.print("S_1:"); if (data > 260 && data < 295) lcd.print("MID"); else if (data < 260) lcd.print("LOW"); else lcd.print("HIGH"); lcd.setCursor(7, 0); lcd.print("Pump:ON"); lcd.setCursor(0, 1); lcd.print("V1:ON"); lcd.setCursor(9, 2); lcd.print("S_2:"); if (data2 > 260 && data2 < 295) lcd.print("MID"); else if (data2 < 260) lcd.print("LOW"); else lcd.print("HIGH"); lcd.setCursor(7, 1); lcd.print("V2:ON"); lcd.setCursor(14, 1); lcd.print("V3:OFF"); sum += data; sum2 += data2; delay(1000); } average = sum / 10; average2 = sum2 / 10; if (average > voltage || average2 > voltage) { digitalWrite(pump, LOW); digitalWrite(valve, LOW); digitalWrite(valve2, LOW); } sum = 0; sum2 = 0; lcd.clear(); }
It seams not going throw the whole conditions.