Slack Webhook - Getting Invalid_Payload
22,685
Solution 1
var payload= {"text":"This is via an integration from Me - It is a test"}
payload = JSON.stringify(payload)
I had forgot to stringify the JSON I was creating. Stupid Me.
Solution 2
This worked for me
var payload = {"text":"Message to be sent"}
payload = JSON.stringify(payload);
request.post({url: url, body: payload},function(err,data){
console.log(data.body);
})
Solution 3
My guess is that you are missing the Content-type: application/json
header. Then it doesn't recognize the json you are sending as json correctly.
You could try:
var request = require('request')
var webhook = "https://hooks.slack.com/services/CUSTOMLINK"
var payload={"text":"This is via an integration from Me - It is a test"}
var headers = {"Content-type": "application/json"}
request.post({url: webhook, payload: payload, headers: headers}, function(err, res){
if(err){console.log(err)}
if(res){console.log(res.body)}
})
Check the "Send it directly in JSON" here for reference
Solution 4
var request = require('request');
var apiurl = webhookurl;
var payload= {
username:'myusername',
text:'test'
}
payload = JSON.stringify(payload);
request.post(
{
url:apiurl,
form:payload
}, function (err, result, body) {
if(err) {
return err;
} else {
console.log(body);
}
});
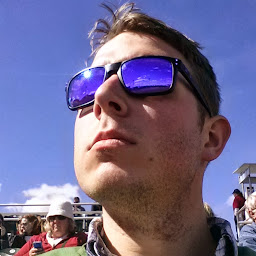
Author by
KJ Carlson
Updated on July 18, 2022Comments
-
KJ Carlson almost 2 years
I am attempting to set up a webhook to Slack, but am getting an Error message of "Invalid_Payload"
I've looked through Stack, Slack, and Github... but cant' find the answer I seek.
"CustomLink" in there for privacy, actual link is begin used.
CODE:
var request = require('request') var webhook = "https://hooks.slack.com/services/CUSTOMLINK" var payload={"text":"This is via an integration from Me - It is a test"} request.post({url: webhook, payload: payload}, function(err, res){ if(err){console.log(err)} if(res){console.log(res.body)} })
ERROR:
invalid_payload