slice shift like function in go lang
Solution 1
For example,
package main
import "fmt"
func main() {
s := []int{2, 3, 5, 7, 11, 13}
fmt.Println(len(s), s)
for len(s) > 0 {
x := s[0] // get the 0 index element from slice
s = s[1:] // remove the 0 index element from slice
fmt.Println(x) // print 0 index element
}
fmt.Println(len(s), s)
}
Output:
6 [2 3 5 7 11 13]
2
3
5
7
11
13
0 []
References:
The Go Programming Language Specification: For statements
Addendum to answer edit to question:
Declare x
,
package main
import "fmt"
func main() {
s := []int{2, 3, 5, 7, 11, 13}
fmt.Println(len(s), s)
for len(s) > 0 {
var x int
x, s = s[0], s[1:]
fmt.Println(x)
}
fmt.Println(len(s), s)
}
Output:
6 [2 3 5 7 11 13]
2
3
5
7
11
13
0 []
You can copy and paste my code for any slice type; it infers the type for x
. It doesn't have to be changed if the type of s
changes.
for len(s) > 0 {
x := s[0] // get the 0 index element from slice
s = s[1:] // remove the 0 index element from slice
fmt.Println(x) // print 0 index element
}
For your version, the type for x
is explicit and must be changed if the type of s
is changed.
for len(s) > 0 {
var x int
x, s = s[0], s[1:]
fmt.Println(x)
}
Solution 2
Just a quick explanation on how we implement shift-like functionality Go. It's actually a very manual process. Take this example:
catSounds := []string{"meow", "purr", "schnurr"}
firstValue := stuff[0] // meow
catSounds = catSounds[1:]
On the first line, we create our slice.
On the second line we get the first element of the slice.
On the third line, we re-assign the value of catSounds
to everything currently in catSounds
after the first element (catSounds[1:]
).
So given all that, we can condense the second and third lines with a comma for brevity:
catSounds := []string{"meow", "purr", "schnurr"}
firstValue, catSounds := catSounds[0], catSounds[1:]
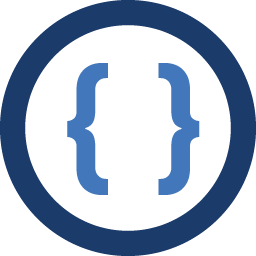
Admin
Updated on June 15, 2022Comments
-
Admin almost 2 years
how array shift function works with slices?
package main import "fmt" func main() { s := []int{2, 3, 5, 7, 11, 13} for k, v := range s { x, a := s[0], s[1:] // get and remove the 0 index element from slice fmt.Println(a) // print 0 index element } }
I found an example from slice tricks but can't get it right.
https://github.com/golang/go/wiki/SliceTricks
x, a := a[0], a[1:]
Edit can you please explain why x is undefined here?
Building upon the answer and merging with SliceTricks
import "fmt" func main() { s := []int{2, 3, 5, 7, 11, 13} fmt.Println(len(s), s) for len(s) > 0 { x, s = s[0], s[1:] // undefined: x fmt.Println(x) // undefined: x } fmt.Println(len(s), s) }
-
Admin about 7 yearsThanks Peter... didn't know for could be used like this, very similar to while loop
-
ardnew almost 3 yearsboth
meow
andpurr
are tocat
asscnurr
is to .....???? If I ever came home to my cat uttering aschnurr
, I'm really not sure how I'd react. Because, quite suddenly, I'd then be aware that some creature-formerly-known-as-cat (possibly unknown to science, folklore, existential dimension, etc.) has been snuggling on my lap for years. From an ethical standpoint, you should really add a disclaimer> CAUTION: READER DISCRETION ADVISED
. -
Roshambo almost 3 years
schnurr
is German forpurr
. coincidentally (or perhaps not)schnurrbart
is German for mustache. -
Julius Žaromskis about 2 years^ the things I learn on stackoverflow :D