SlickGrid select editor
Solution 1
A similar queations was asked here (this one is however not slickgrid tagged).
I did make a SelectEditor, with a flexible range of options depending on the column we are in. The reason of thinking here is that the datatype of the value you edit in a column will determine the valid choices for this field.
In order to do this you can add an extra option to your column definitions (e.g. called options) like this:
var columns = [
{id:"color", name:"Color", field:"color", options: "Red,Green,Blue,Black,White", editor: SelectCellEditor}
{id:"lock", name:"Lock", field:"lock", options: "Locked,Unlocked", editor: SelectCellEditor},
]
and access that using args.column.options in the init method of your own SelectEditor object like this:
SelectCellEditor : function(args) {
var $select;
var defaultValue;
var scope = this;
this.init = function() {
if(args.column.options){
opt_values = args.column.options.split(',');
}else{
opt_values ="yes,no".split(',');
}
option_str = ""
for( i in opt_values ){
v = opt_values[i];
option_str += "<OPTION value='"+v+"'>"+v+"</OPTION>";
}
$select = $("<SELECT tabIndex='0' class='editor-select'>"+ option_str +"</SELECT>");
$select.appendTo(args.container);
$select.focus();
};
this.destroy = function() {
$select.remove();
};
this.focus = function() {
$select.focus();
};
this.loadValue = function(item) {
defaultValue = item[args.column.field];
$select.val(defaultValue);
};
this.serializeValue = function() {
if(args.column.options){
return $select.val();
}else{
return ($select.val() == "yes");
}
};
this.applyValue = function(item,state) {
item[args.column.field] = state;
};
this.isValueChanged = function() {
return ($select.val() != defaultValue);
};
this.validate = function() {
return {
valid: true,
msg: null
};
};
this.init();
}
Solution 2
You can slightly modify the above SelectCellEditor to create different select options for each cell.
function SelectCellEditor(args) {
.....
// just to get the DOM element
this.getInputEl = function() {
return $input;
};
}
Now it is easy to create a dynamic dropdown editor.
function DynamicSelectCellEditor(args) {
// 1: if you already have a select list for individual cells
args.columns.options = optionsList[args.item.id] // or any custom logic
return new Slick.Editors.SelectCellEditor(args);
/* OR */
// 2: if data needs to be fetched from the server
var editor = new Slick.Editors.SelectCellEditor(args),
$select = editor.getInputEl();
$select.html("<option>Loading...</option>");
$.ajax({ }).done(function(list) {
// Update select list
$select.html(newHtml);
});
return editor;
}
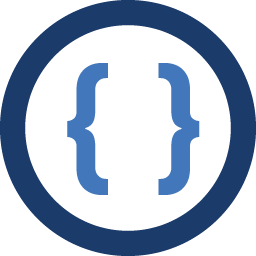
Admin
Updated on July 24, 2022Comments
-
Admin almost 2 years
I want to make a dynamically populated html select for a select cell. I extract some information from a database which is different for every row item. The problem is that the editor loses the initial data and I don't know how to keep some data for a specific cell. Has someone done this before?
function StandardSelectCellEditor($container, columnDef, value, dataContext) { var $input; var $select; var defaultValue = value; var scope = this; this.init = function() { $input = $("<INPUT type=hidden />"); $input.val(value); } $input.appendTo($container); $select = $("<SELECT tabIndex='0' class='editor-yesno'>"); jQuery.each(value, function() { $select.append("<OPTION value='" + this + "'>" + this + "</OPTION></SELECT>"); }); $select.append("</SELECT>"); $select.appendTo($container); $select.focus(); }; this.destroy = function() { //$input.remove(); $select.remove(); }; this.focus = function() { $select.focus(); }; this.setValue = function(value) { $select.val(value); defaultValue = value; }; this.getValue = function() { return $select.val(); }; this.isValueChanged = function() { return ($select.val() != defaultValue); }; this.validate = function() { return { valid: true, msg: null }; }; this.init(); };
-
Matthijs over 13 yearsDo you really need different select options for each individual field ? If so you may consider coding the value if each field itself as an object having the value and its options (e.g. "Blue|Red,Green,Blue,Black,White". You need an extra CellFormatter to show the value, and you can change the CellEditor code to get the options from the value after the "|" sign. Take care to write back the value including the option list after edit is done in order not to lose this information ...