SMTP mail error in CodeIgniter : server might not be configured to send mail
14,219
I think you need to load the email library in constructor like
$this->load->helper(array('email')); $this->load->library(array('email'));
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
class Dispatch extends MY_Controller
{
function __construct() {
parent::__construct();
$this->load->helper('url');
$this->load->helper(array('form', 'url'));
$this->load->library('form_validation');
$this->load->library('session');
$this->load->helper(array('email'));
$this->load->library(array('email'));
}
public function sendEmail()
{
$config = array(
'protocol' => 'smtp',
'smtp_host' => 'smtp.googlemail.com',
'smtp_port' => '587',
'smtp_user' => '[email protected]',
'smtp_pass' => 'XXXXXXX',
'mailtype' => 'html',
'charset' => 'iso-8859-1'
);
$this->load->library('email', $config);
$this->email->set_newline("\r\n");
$this->email->set_mailtype("html");
$message2 = '
<html>
<head>
<title>Dispatch</title>
</head>
<body>
Test Mail
</body>
</html>
';
$subject="Test Email";
$message=$message2;
$this->email->from('[email protected]');
$this->email->to([email protected]);
$this->email->subject($subject);
$this->email->message($message);
if($this->email->send())
{
echo "<script type='text/javascript'>alert('Email Send Success');</script>";
redirect('/Dispatch/', 'refresh');
}
else
{
show_error($this->email->print_debugger());
}
}
}
?>
I am also facing same problem but when I try to load library in constructor the email send success.this is work for me hope this will help you too.
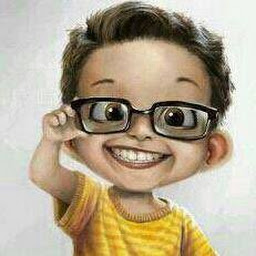
Author by
Sunil Kashyap
My name is Sunil Kashyap, I am a Full Stack Web Developer specialized in Javascript and Web Technologies. Currently working and living in Nagpur, India. https://sunilkashyap.com https://dev-sunil.com
Updated on June 04, 2022Comments
-
Sunil Kashyap almost 2 years
I am trying to send SMTP email using CodeIgniter, but I'm getting error in response ...
"The following SMTP error was encountered: 0 Unable to send email using PHP SMTP. Your server might not be configured to send mail using this method."
User-Agent: CodeIgniter Date: Tue, 13 Dec 2016 07:06:56 +0000 From: "Blabla" <xxxxxxxxxxxxxx> Return-Path: <xxxxxxxxxxxxxxxxxxx> To: xxxxxxxxxxxx Reply-To: "Explendid Videos" <xxxxxxxxxxxxxxxxxx> Subject: =?utf-8?Q?=54=68=69=73=20=69=73=20=61=6E=20=65=6D=61=69=6C=20=74=65=73=74?= X-Sender: [email protected] X-Mailer: CodeIgniter X-Priority: 3 (Normal) Message-ID: <[email protected]> Mime-Version: 1.0 Content-Type: multipart/alternative; boundary="B_ALT_584f9e1023f99" This is a multi-part message in MIME format. Your email application may not support this format. --B_ALT_584f9e1023f99 Content-Type: text/plain; charset=utf-8 Content-Transfer-Encoding: 8bit It is working. Great! --B_ALT_584f9e1023f99 Content-Type: text/html; charset=utf-8 Content-Transfer-Encoding: quoted-printable It is working. Great! --B_ALT_584f9e1023f99--
Code is
$ci = get_instance(); $ci->load->library('email'); $config = array(); $config['protocol'] = "smtp"; $config['smtp_host'] = "xxxxx"; $config['smtp_port'] = 25; $config['smtp_user'] = "xxxxx"; $config['smtp_pass'] = "xxxxx"; $config['charset'] = "utf-8"; $config['mailtype'] = "html"; $config['newline'] = "\r\n"; $ci->email->initialize($config); $ci->email->from('xxxxx', 'Blabla'); $list = array('xxxxx'); $ci->email->to($list); $this->email->reply_to('xxxxx', 'Explendid Videos'); $ci->email->subject('This is an email test'); $ci->email->message('It is working. Great!'); if($ci->email->send()){echo "send";}else{echo "error ";print_r($ci->email->print_debugger());}