split string in javascript, reactjs
You have split your type
, but havent made use of them yet.
As you have split your type
, you would get answer_array
with a length of 3
containing ["Brown", "D", "JP"]
const answer_array = answer.split(',');
Next you are updating your state with the updated answer count. You are performing the below
const updatedAnswersCount = update(this.state.answersCount, {
[answer]: {$apply: (currentValue) => currentValue + 1},
});
Here answer
contains "Brown,D,JP"
. Since you want to update each of it by +1, lets loop over the split value and update it.
let updatedAnswersCount = null;
answer_array.forEach((key) => {
updatedAnswersCount = update(this.state.answersCount, {
[answer]: {$apply: (currentValue) => currentValue + 1},
});
}
Here, i'm assuming that your type is unique. Meaning Brown
/D
/JP
is present only for this answer and not for anything else. So we are assuming all will have same value.
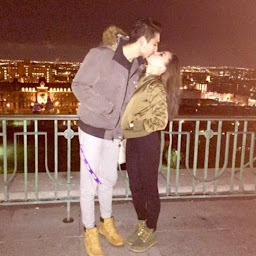
Irfan
Updated on July 09, 2022Comments
-
Irfan almost 2 years
so I'm developing a quiz application sort of, so this is my initial state of the app when it first launch, I also have quizApplication.js component store all the question and answers,
{ question: "I am task oriented in order to achieve certain goals", answers: [ { type: "Brown,D,JP", content: "Hell Ya!" }, { type: " ", content: "Nah" } ] },
and here is my function to set the user answer
setUserAnswer(answer) { if (answer.trim()) { const answer_array = answer.split(','); const updatedAnswersCount = update(this.state.answersCount, { [answer]: {$apply: (currentValue) => currentValue + 1}, }); this.setState({ answersCount: updatedAnswersCount, answer: answer }); } }
I also have a AnswerOption component like so
function AnswerOption(props) { return ( <AnswerOptionLi> <Input checked={props.answerType === props.answer} id={props.answerType} value={props.answerType} disabled={props.answer} onChange={props.onAnswerSelected} /> <Label className="radioCustomLabel" htmlFor={props.answerType}> {props.answerContent} </Label> </AnswerOptionLi> ); }
So what im try to do is that whenever the user click on HellYa! it will increment "Brown" and "D" and "JP" by +1, but right now it gives me a new answersCount value as Brown,D,JP: null, so how should I achieve this? Many thanks!
-
Fareed Alnamrouti over 6 yearsyour question still not clear where is the implementation of the update function ? also where i the container where you use the AnswerOption component ?
-
Fareed Alnamrouti over 6 yearsalso if you are using react 16 according to the documentation you should not relay on current state to set the next state, because the setState function now is Asynchronous and multiple setState can be patched together under the hood, read here: reactjs.org/docs/…
-
-
Irfan over 6 yearsforeach makes sense, but its still giving me new value for answerCount like Brown,D,JP: null, my init state in contructor is like this answersCount: { Green: 0, Brown: 0, Blue: 0, Red: 0, A: 0, B: 0, C: 0, D: 0, EI: 0, SN: 0, TF: 0, JP: 0 },
-
Irfan over 6 yearsok, so for the first part i did it without passing that key param, now i changed the key param to answer, when i use console.log(updatedAnswersCount) it will give me what i want by Brown update 1 and D update 1 etc. but when i go back to my state, answersCount only update the last value JP by 1, i dont know if you know what i mean