Spring injecting a static (global) singleton
Solution 1
There are some difference between Singleton as a design pattern and Spring's singleton facility. Singleton as a design pattern will ensure you have one object of class defined per Class Loader. Spring's singleton facility (and approach), in contrast, will define one instance per Spring Context.
In this case you can utilize your getInstance()
method to be used by Spring to grab your object instance:
<bean id="configurator" class="com.me.myapp.Configurator" factory-method="getInstance">
</bean>
With Spring the singleton
bean scope is the default therefore you don't need to define it.
If you want to use configurator
as a Spring bean you will have to inject it in other objects, not use getInstance()
to grab it. So in other Spring beans use @Autowired or define reference to bean through xml file. If you don't reorganize usage of configurator
in other classes, there will no be difference, Spring will instantiate your class, but you will use it as before.
Also I saw that you have an error in designing your singleton. Your getInstance()
method should be public, and other methods should not be static. In the example you've used you should use Singleton like this:
Configurator.getInstance().someMethod()
In this case you actually just use the Singleton class, without instantiating any objects! See wikipedia on Singleton (with Java example) for more information on the Singleton design pattern and how to use it.
NOTE: It's worth knowing and attempting to use
Configurator
as a Singleton and make use Spring's singleton facility. If you do this the benefits will be that you can
- Remove the
getInstance()
method - Make your constructor public
- Let the Spring instantiate that single object.
Solution 2
Beans are singleton by default. You can find this/more information out via the spring website.
You shouldn't instantiate a new Configurator in getInstance because it won't refer to the spring loaded bean and that could cause some serious issues. You can wire this bean in and then leave it alone, it won't be null because you've wired it in (and if it is your program will have failed initialising).
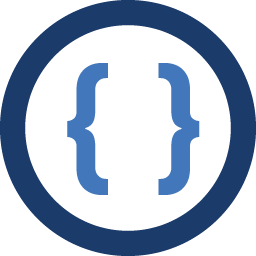
Admin
Updated on October 12, 2020Comments
-
Admin over 3 years
I have a class that looks like so:
public class Configurator { private static Configurator INSTANCE = null; private int maxRange = 1; // many other properties; each property has a default value private static synchronized Configurator getInstance() { if(INSTANCE == null) return new Configurator(); return INSTANCE; } public static int getMaxRange() { getInstance().maxRange; } public static void setMaxRange(int range) { getInstance().maxRange = range; } // Getters and setters for all properties follow this pattern }
It serves as a global configuration object that can be set on app startup, and then is used by dozens of classes throughout the project:
// Called at app startup to configure everything public class AppRunner { Configurator.setMaxRange(30); } // Example of Configurator being used by another class public class WidgetFactory { public void doSomething() { if(Configurator.getMaxRange() < 50) // do A else // do B } }
I'm now importing this code into a Spring project, and am trying to configure my Sprinig XML (beans). My guess is that I could define a lone
Configurator
bean like so (or something similar):<bean id="configurator" class="com.me.myapp.Configurator" scope="singleton"> <property name="maxRange" value="30"/> <!-- etc., for all properties --> </bean>
That way, when
WidgetFactory#doSomething
executes, Spring will have already loaded theConfigurator
class and configured it ahead of time.Is it correct for me to set the
scope="singleton"
, or does this not matter? Am I setting the static properties correctly? Is there anything else I need to do or consider here? Thanks in advance. -
Admin over 11 yearsThanks @partlov (+1) - would I need to make my
getInstance()
method public, or can Spring handle the fact that it's private? -
Bob Flannigon over 11 yearsYou don't need it (getInstance())
-
Freddy almost 3 yearsMaybe leave constructor private, use getInstane() to inject a singleton into spring, this way, we can autowire the singleton instance and don't need to call 'getInstance' everytime.